An idea could be to draw the lineplot with a fixed color, and use a scatterplot for colorizing:
import matplotlib.pyplot as plt
import seaborn as sns
import numpy as np
import pandas as pd
df = pd.DataFrame({'DATETIME': pd.date_range('20160101', periods=1000, freq='D'),
'TOTALDEMAND': np.random.randint(-10, 11, 1000).cumsum() + 30000})
df['SEASON'] = pd.Categorical.from_codes((df.DATETIME.dt.month - 1) // 3, ['winter', 'spring', 'summer', 'autumn'])
plt.figure(figsize=(15, 6))
ax = sns.lineplot(data=df, x='DATETIME', y='TOTALDEMAND', color='black')
sns.scatterplot(data=df, x='DATETIME', y='TOTALDEMAND', s=20, alpha=0.2, hue='SEASON', ax=ax)
sns.despine()
plt.show()
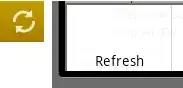