The best way to achieve this is to reshape your dataset long, and then use position_dodge
to separate the bars corresponding to different times. So:
library(ggplot2)
library(tidyr)
dat %>%
pivot_longer(cols=-c(Id,Time)) %>%
ggplot(aes(x=name, y=value, fill=Time, group=Time)) +
stat_summary(geom="col",width=0.8,position=position_dodge()) +
stat_summary(geom="errorbar",width=0.2,position=position_dodge(width=0.8))
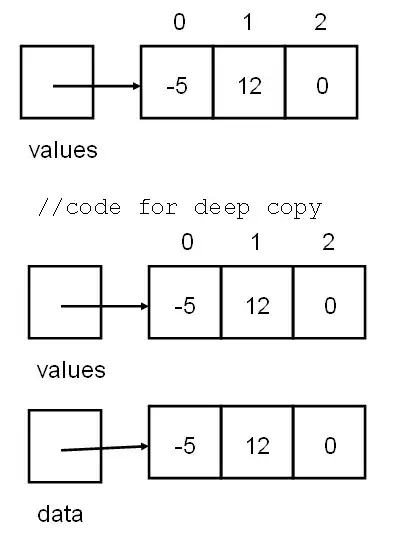
Consider also adding the data points for extra transparency. It will be easier for your readers to understand the data and judge its meaning if they can see the individual points:
dat %>%
pivot_longer(cols=-c(Id,Time)) %>%
ggplot(aes(x=name, y=value, fill=Time, group=Time)) +
stat_summary(geom="col",width=0.8,position=position_dodge()) +
stat_summary(geom="errorbar",width=0.2,position=position_dodge(width=0.8)) +
geom_point(position = position_dodge(width=0.8))
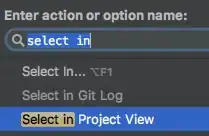
In response to the comment requesting re-ordering of factors and specifying colours, I have added a line to transform Time
to a factor while specifying the order, specifying the limits
for the x-axis to set the order of the groups, and using scale_fill_manual
to set the colours for the bars.
dat %>%
mutate(Time = factor(Time, levels=c("pre", "post"))) %>%
pivot_longer(cols=-c(Id,Time)) %>%
ggplot(aes(x=name, y=value, fill=Time, group=Time)) +
stat_summary(geom="col",width=0.8,position=position_dodge()) +
stat_summary(geom="errorbar",width=0.2,position=position_dodge(width=0.8)) +
geom_point(position = position_dodge(width=0.8)) +
scale_x_discrete(limits=c("Tyr1", "Tyr2", "Baseline")) +
scale_fill_manual(values = c(`pre`="lightgrey", post="darkgrey"))
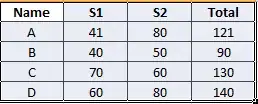
To understand what is happening with the reshape, the intermediate dataset looks like this:
> dat %>%
+ pivot_longer(cols=-c(Id,Time))
# A tibble: 18 x 4
Id Time name value
<int> <chr> <chr> <dbl>
1 1 pre Baseline 0.536
2 1 pre Tyr1 0.172
3 1 pre Tyr2 0.141
4 2 post Baseline 0.428
5 2 post Tyr1 0.046
6 2 post Tyr2 0.084
7 3 pre Baseline 0.077
8 3 pre Tyr1 0.015
9 3 pre Tyr2 0.063
10 4 post Baseline 0.2
11 4 post Tyr1 0.052
12 4 post Tyr2 0.041
13 5 pre Baseline 0.161
14 5 pre Tyr1 0.058
15 5 pre Tyr2 0.039
16 6 post Baseline 0.219
17 6 post Tyr1 0.059
18 6 post Tyr2 0.05