If I didn't misunderstood the question, you can wrap your content with a ModalBottomSheetLayout
.
ModalBottomSheetLayout(
sheetState = modalBottomSheetState,
sheetContent = {
BottomSheetContent()
}
) {
Scaffold(...)
}
The result would be something like this:
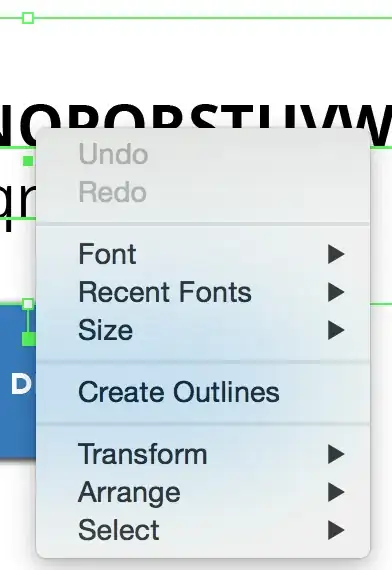
It's not totally related to your question, but if you want to avoid the half-expanded state, you can do the following:
- Declare the function below:
@ExperimentalMaterialApi
suspend fun ModalBottomSheetState.forceExpand() {
try {
animateTo(ModalBottomSheetValue.Expanded)
} catch (e: CancellationException) {
currentCoroutineContext().ensureActive()
forceExpand()
}
}
- In your Bottom Sheet declaration, do the foollowing:
val modalBottomSheetState = rememberModalBottomSheetState(
initialValue = ModalBottomSheetValue.Hidden,
confirmStateChange = {
it != ModalBottomSheetValue.HalfExpanded
}
)
- Call the following to show/hide the Bottom Sheet:
coroutineScope.launch {
if (modalBottomSheetState.isVisible) {
modalBottomSheetState.hide()
} else {
modalBottomSheetState.forceExpand()
}
}