If I understand well, this is an example to do it programmatically... Declare button under your controller class:
let myButton: UIButton = {
let b = UIButton()
b.backgroundColor = .black
b.setTitle("Tap me!", for: .normal)
b.setTitleColor(.white, for: .normal)
b.titleLabel?.font = .systemFont(ofSize: 17, weight: .regular)
b.layer.cornerRadius = 12
b.clipsToBounds = true
b.translatesAutoresizingMaskIntoConstraints = false
return b
}()
Now in viewDidLoad add target and set constraints:
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .white
myButton.addTarget(self, action: #selector(callSeetController), for: .touchUpInside)
view.addSubview(myButton)
myButton.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true
myButton.centerYAnchor.constraint(equalTo: view.centerYAnchor).isActive = true
myButton.heightAnchor.constraint(equalToConstant: 50).isActive = true
myButton.widthAnchor.constraint(equalToConstant: 200).isActive = true
}
After that call the func to present an set sheet attributes:
@objc fileprivate func callSeetController() {
let detailViewController = SecondController()
let nav = UINavigationController(rootViewController: detailViewController)
nav.isModalInPresentation = true // disable swipe down
if let sheet = nav.sheetPresentationController {
sheet.detents = [.large()]
}
let image = UIImage(systemName: "x.circle")
let dismiss = UIBarButtonItem(image: image, primaryAction: .init(handler: { [weak self] _ in
if let sheet = nav.sheetPresentationController {
sheet.animateChanges {
self?.dismiss(animated: true, completion: nil)
}
}
}))
detailViewController.navigationItem.rightBarButtonItem = dismiss
detailViewController.navigationController?.navigationBar.tintColor = .white
present(nav, animated: true, completion: nil)
}
The result:
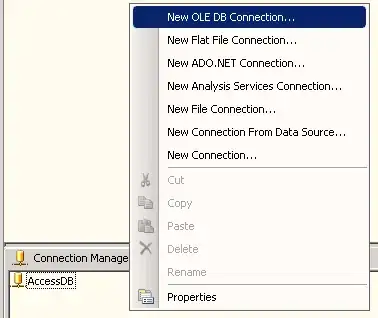