You can mitigate the effect of the black transition line in Python/OpenCV/Skimage by antialiasing the alpha channel as follows:
- Read the image with alpha
- Extract the bgr base image
- Extract the alpha channel
- Gaussian blur the alpha channel (choose the blur amount so as to match the black transition)
- Stretch the dynamic range of the alpha channel so that 255 -> 255 and mid-gray goes to 0 and save as mask. (Choose the mid-gray level to mitigate further)
- Put the resulting mask image into the alpha channel of the bgr image
- Save the results
Input:
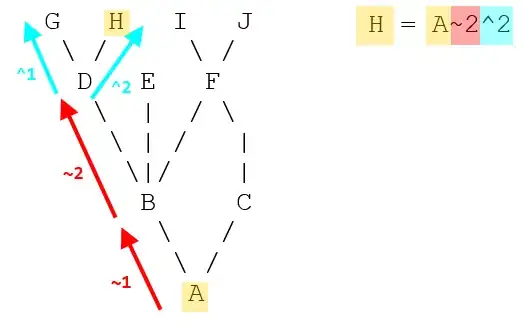
import cv2
import numpy as np
import skimage.exposure
# load image with alpha
img = cv2.imread('room.png', cv2.IMREAD_UNCHANGED)
# extract only bgr channels
bgr = img[:, :, 0:3]
# extract alpha channel
alpha = img[:, :, 3]
# apply Gaussian blur to alpha
blur = cv2.GaussianBlur(alpha, (0,0), sigmaX=5, sigmaY=5, borderType = cv2.BORDER_DEFAULT)
# stretch so that 255 -> 255 and 192 -> 0
mask = skimage.exposure.rescale_intensity(blur, in_range=(192,255), out_range=(0,255)).astype(np.uint8)
# put mask into alpha channel
result = np.dstack((bgr, mask))
# save result
cv2.imwrite('room_new_alpha.png', result)
# display result, though it won't show transparency
cv2.imshow("bgr", bgr)
cv2.imshow("alpha", alpha)
cv2.imshow("mask", mask)
cv2.imshow("result", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
Result:
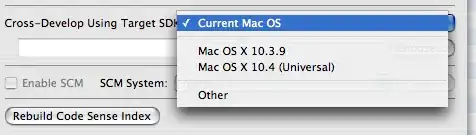