Not sure if there is a way to change the window's size, run mode, or whatever it is called from the batch script itself. It really doesn't matter since you can either control it via shortcut, or just avoid the situation all together.
If you look at the comments on your question, Compo mentioned using a shortcut - this is the most direct answer to your question. Do the following to create desired shortcut:
- Create a shortcut by opening windows explorer and navigating to where your batch file is.
- Start a drag-n-drop operation by grabbing the batch file with the mouse and moving the mouse off of the file, but DO NOT let go yet. Hold down the CTRL+Shift and the icon you are dragging, or the text under it, will change to indicate you are about to create a link (A.K.A. shortcut). Letting go in the same folder will work, but you may want to move the shortcut later. Alternatively, you could try bringing up a context menu on the batch file by moving the mouse over it and using the secondary click, and then select "create shortcut", which should be found fairly far done on the context menu.
- Select the newly created shortcut with the mouse and on the keyboard type Alt+Enter - a properties window should appear. If for some reason that fails. you can use the mouse to bring up a context menu on the newly created shortcut (same way as described in step 2) and select "Properties" (Should be at bottom of context menu).
- Go to the "Shortcut" tab in the "Properties" windows, select "Normal windows" from "Run:" section, and click the "OK" button (See image below).
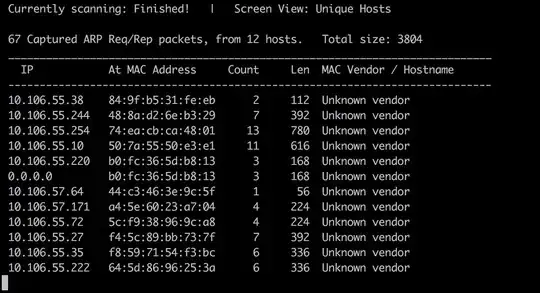
The resulting icon can be moved to the desktop or pinned to the taskbar by dragging to the taskbar.
Or instead, you could rewrite your code so it doesn't ask any questions and just does the job. If you only have 2 choices, why not check which is current, change to the other, and report which one is now the current option?
The following code:
- Verifies that copies of both the senpai and nakano configs exist before making any changes.
- Checks if the file "Nakano.Active" exist, if so then it does the code to switch to senpai, else it assumes senpai is active and does the code to switch to nakano.
- Replaces the current "config.yml" file with the desired configuration.
- Deletes the current "{Mode}.Active" file, "Nakano.Active" or "Senpai.Active", as needed.
- Creates a new Active file, or flag, to indicate the current mode. That is , creates "Senpai.Active" or "Nakano.Active", as needed.
- Echos/reports out what mode it just switch to.
- Waits for 2.25 seconds, giving you the time to see what mode it switched to. Change the 225 to the number of centiseconds you want the code to wait before continuing.
- Executes EXIT which closes the window.
@ECHO OFF
IF NOT EXIST "config - senpai.yml" GOTO :DoExit
IF NOT EXIST "config - nakano.yml" GOTO :DoExit
IF EXIST Nakano.Active (
COPY /Y "config - senpai.yml" "config.yml" >NUL
DEL Nakano.Active 2>NUL
ECHO;Senpai >Senpai.Active
ECHO;Senpai Mode
) ELSE (
COPY /Y "config - nakano.yml" "config.yml" >NUL
DEL Senpai.Active 2>NUL
ECHO;Nakano >Nakano.Active
ECHO;Nakano Mode
)
REM Pause for 2.25 seconds
SET Centiseconds=225
SET "NOWTIME=%TIME:00.=%"
SET "NOWTIME=%NOWTIME:.=%"
FOR /F "TOKENS=1-3 DELIMS=:" %%G IN ("%NOWTIME::0=:%") DO SET /A "StartCentiseconds=(%%G*60+%%H)*6000+%%I"
SET /A EndCentiseconds=StartCentiseconds+Centiseconds
:GetNow
SET "NOWTIME=%TIME:00.=%"
SET "NOWTIME=%NOWTIME:.=%"
FOR /F "TOKENS=1-3 DELIMS=:" %%G IN ("%NOWTIME::0=:%") DO SET /A "NowCentiseconds=(%%G*60+%%H)*6000+%%I"
IF %NowCentiseconds% LSS %StartCentiseconds% SET /A NowCentiseconds=NowCentiseconds+8640000
IF %NowCentiseconds% LSS %EndCentiseconds% GOTO :GetNow
:DoExit
EXIT