I implemented a sample which can give you a kick-off in your implementation:
@Composable
fun CanvasWithHole(
holeXPosition: Float,
holeYPosition: Float,
holeRadius: Float
) {
androidx.compose.foundation.Canvas(
modifier = Modifier.fillMaxSize(),
onDraw = {
drawIntoCanvas { canvas ->
val w = drawContext.size.width
val h = drawContext.size.height
drawImageWithHole(
canvas.nativeCanvas,
w, h, holeXPosition, holeYPosition, holeRadius,
)
}
}
)
}
fun drawImageWithHole(
canvas: Canvas,
w: Float,
h: Float,
holeXPosition: Float,
holeYPosition: Float,
holeRadius: Float
) {
val bitmap = Bitmap.createBitmap(
w.toInt(), h.toInt(), Bitmap.Config.ARGB_8888
).apply {
eraseColor(Color.TRANSPARENT)
}
val canvasBitmap = Canvas(bitmap)
val eraser = Paint().apply {
color = Color.TRANSPARENT
xfermode = PorterDuffXfermode(PorterDuff.Mode.CLEAR)
}
canvasBitmap.drawColor(Color.parseColor("#cc328fa8"))
canvasBitmap.drawCircle(holeXPosition, holeYPosition, holeRadius, eraser)
canvas.drawBitmap(bitmap, 0f, 0f, null)
}
And here is how you can use it:
@Composable
fun MyScreen() {
Box {
ContentScreen()
CanvasWithHole(
100f,
100f,
400f,
)
}
}
Here's the result:
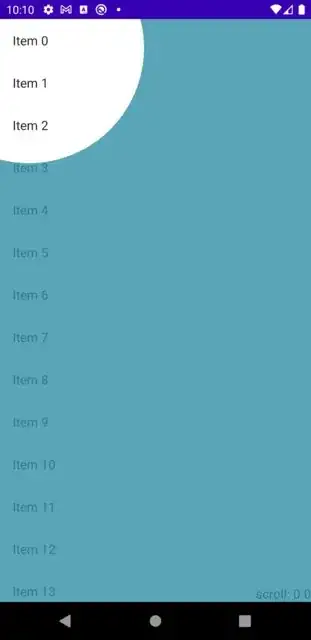