when I initialize one object to another in java
I do not know what you mean by "to another". But you initialized only a single object.
First line
Regarding your first line:
A obj1=new A("apple");
A obj1
declares a reference variable, giving it a name obj1
, and specifies that this var will hold a reference to any object of type A
. This reference variable is initially null.
Calling new A("apple")
constructs an object some place in memory. The result, the return value, of this code is a reference to the new object.
The =
assigns that reference to be the content of the variable named obj1
.
You can think of a reference variable as containing the address in memory where the beginning of that object's block of allocated memory resides. In Java we never see the literal contents of a reference variable. But we know we can reach the object via that reference variable.
In our daily work of programming in Java, we may generally think of obj1
as being the object. But actually obj1
is a way to find the object, a tether attached to the object, a line we can follow to access the object somewhere else in memory.
Second line
Regarding your second line:
A obj2=obj1;
First you declare a new variable named obj2
to hold a reference to an object of type A
. Then you copied the reference from obj1
and put that copy into obj2
.
You are left with:
- A single object.
- Two references to that single object.
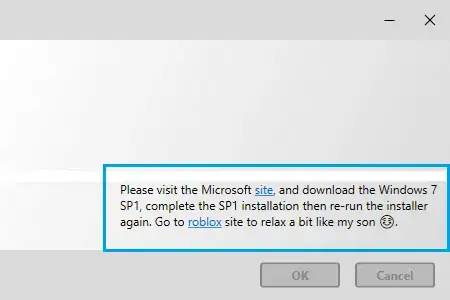