Here's an example of how you can write your code. You can simulate this on a single site like I did. On my site, I created 3 folders:
- example1
- example2
- exampleapi
Under each of them, I create an index.php file. Let's look at them.
yoursite.com/exampleapi/index.php
<?php
header("Content-Type: application/text");
echo 'Titanic vs. Iceberg';
The output of this is plaintext
Titanic vs. Iceberg
We'll call this API from example1.
yoursite.com/example1/index.php
This site's code will have it's own data and
will pull data from exampleapi/index.php like so:
<?php
// call exampleapi
$url = 'https://yoursite.com/exampleapi/index.php';
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
$response = curl_exec($curl);
curl_close($curl);
// show data
header("Content-Type: application/json");
$data = [
'name' => 'John',
'age' => 25,
'data_from_exampleapi' => $response
];
echo json_encode($data);
Output of this code will be
{"name":"John","age":25,"data_from_exampleapi":"Titanic vs. Iceberg"}
We'll call this from example2.
yoursite.com/example2/index.php
This will be your webpage. I have simulated a call. When the button is pressed, PHP will send a request to example1/index.php. And that page will send a request to exampleapi/index.php, fetch the data, combine the fetched data with its own data and send it back to example2/index.php
<?php
function fetchInformation()
{
$url = 'https://yoursite.com/example1/index.php';
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
$response = curl_exec($curl);
curl_close($curl);
return $response;
}
?>
<!doctype html>
<body>
<form action="./" method="get">
<p>
When you press this button, PHP will try to get data from
example1/index.php and show information
below
</p>
<input type="submit" value="Get data from API" name="mybutton" id="mybutton">
</form>
<?php
// only if button is pressed, fetch information
if ($_SERVER['REQUEST_METHOD'] === 'GET')
{
if ( ! is_null($_REQUEST['mybutton']) )
{
echo '<h3>Information received</h3>';
$response = fetchInformation();
echo '<p>' . $response . '</p>';
}
}
?>
</body>
When you go to yoursite.com/example2/index.php, you'll see something like this:
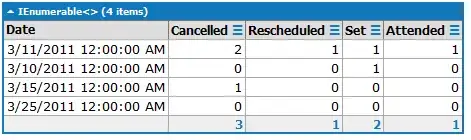
When you press the button, example2/index.php -> calls example1/index.php -> calls exampleapi/index.php. Data goes in reverse and you'll see this kind of an output:
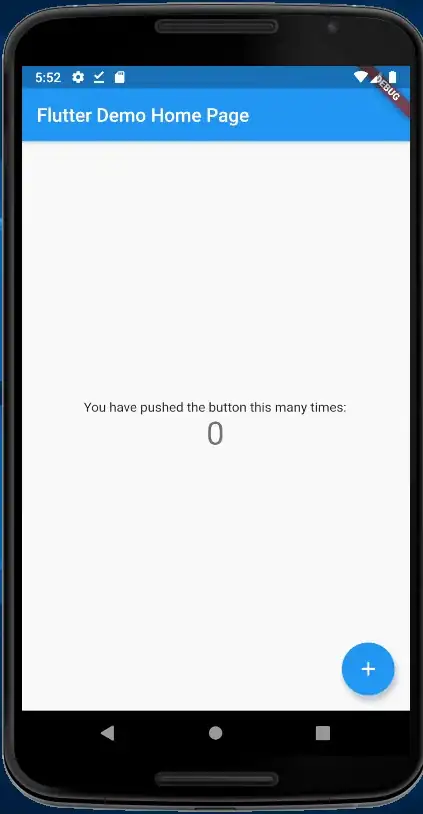
This shows you how you can use PHP one 1 page to call API on another page. If you are in control of that another page, you can tweak code to call API from yet-another-page.