Fast answer:
Now it goes like this:
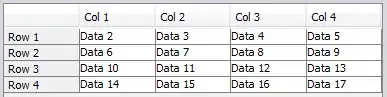
Just to check any number (including 0 and 0.0) is divisible by 2 and/or 3, you should remove your 'while b:' instruction. But I believe you entered these inputs to check numbers in loop... So you want to exit if input is empty, but that will raise error during converting to float.
So, assuming minimal effort to improve this, my advice is to approach that this way:
b=input("enter the number to check: ")
while str(b): #this will check if your number as string is missing
b = float(b)
if b%2==0 and b%3==0 :
print("this number divisable by 2 and 3")
b=input("enter the number to check: ")
elif b%2==0 and b%3!=0 :
print("this number divisable by 2 only")
b=input("enter the number to check: ")
elif b%3==0 and b%2!=0 :
print("this number divisable by 3 only")
b=input("enter the number to check: ")
else:
print("it's not divisable by any one of 2 and 3")
b=input("enter the number to check: ")
Of course, there are a lot other ways how you can improve it...
First just ask once for input if that happens for every if statement, just right after it ends, and show for yourself when condition failed and program ended correctly:
b=input("enter the number to check: ")
while str(b): # You want
b = float(b)
if b%2==0 and b%3==0 :
print("this number divisable by 2 and 3")
elif b%2==0 and b%3!=0 :
print("this number divisable by 2 only")
elif b%3==0 and b%2!=0 :
print("this number divisable by 3 only")
else:
print("it's not divisable by any one of 2 and 3")
b=input("enter the number to check: ")
print("program ended")
There are possible further improvements, like optimization of statements, checking results without double calculations, byte flag check for division by 2, sum of str for big number divided by 3 and so on, if you're interested how I would approach it, feel free to make request in comment :)
@Edit: As 'requested' in comments by @MartijnPjeters, more valid code I would consider as valid, even when there the slowest component there is user requested for input :P
while True:
try:
b = int(input("enter the number to check: "))
except ValueError: #Alternative: b=input... b.isdigit() then int(b)
print("That is not integer, exiting loop")
break
divisible_by_2 = b^1
divisible_by_3 = not b%3
if divisible_by_2 and divisible_by_3:
print("this number divisable by 2 and 3")
elif divisible_by_2:
print("this number divisable by 2 only")
elif divisible_by_3:
print("this number divisable by 3 only")
else:
print("it's not divisable by any one of 2 and 3")
print("program ended")