As a brief context, I am getting raw video data from Zoom SDK as separate Y, U, and V ByteBuffers and trying to convert them to bitmaps. However, I noticed that this conversion is resulting in grayscale bitmap images with green and pink spots [as shown in the screenshot below]
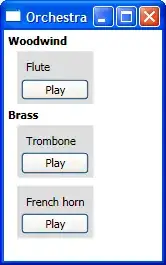
the method Iām using for conversion is the following:
fun ZoomVideoSDKVideoRawData.toBitmap(): Bitmap? {
val width = streamWidth
val height = streamHeight
val yuvBytes = ByteArray(width * (height + height / 2))
val yPlane = getyBuffer()
val uPlane = getuBuffer()
val vPlane = getvBuffer()
// copy Y
yPlane.get(yuvBytes, 0, width * height)
// copy U
var offset = width * height
uPlane.get(yuvBytes, offset, width * height / 4)
// copy V
offset += width * height / 4
vPlane.get(yuvBytes, offset, width * height / 4)
// make YUV image
val yuvImage = YuvImage(yuvBytes, ImageFormat.NV21, width, height, null)
// convert image to bitmap
val out = ByteArrayOutputStream()
yuvImage.compressToJpeg(Rect(0, 0, width, height), 50, out)
val imageBytes = out.toByteArray()
val image = BitmapFactory.decodeByteArray(imageBytes, 0, imageBytes.size)
// return result
return image
}
any idea why the colors of the resulting bitmap are corrupted? Thanks in advance!