TLDR; Your mistake was rendering 3-digits as a whole thing; You should have 3-sprite renderer, each displaying a single digit.
Set in the inspector, an array of Sprites for the digits, ordered properly.
You will use the array as a Dictionary to easily access any digit-sprite by a corresponding number:
[SerializeField, Tooltip("Your number 0-9 sprites should be here, ordered correctly.")]
private Sprite[] numberSprites;
// ......
numberSprites[4] // will output '4' sprite;
Next, rather than have 1 big whole renderer to represent all 3 digits, we can split it into 3 renderer, and expose it to the inspector as an array:
[SerializeField]
private SpriteRenderer[] scoreSpriteRenderers;
Each of this renderer should only render 1 single-digit, something like this: 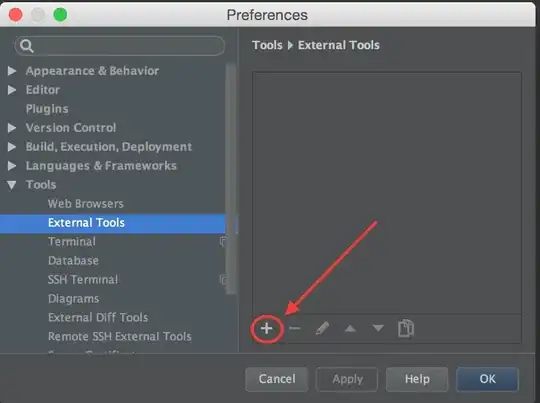
Now we can easily set-up a loop, to loop through each number in the score, and change the sprite based on the number.
The full result:
[SerializeField, ToolTip("Your number 0-9 sprites should be here, ordered correctly.")]
private Sprite[] numberSprites;
[SerializeField]
private SpriteRenderer[] scoreSpriteRenderers;
private int scoreCount;
// Converts the '123' into a {1, 2, 3}
// See: https://stackoverflow.com/questions/829174/is-there-an-easy-way-to-turn-an-int-into-an-array-of-ints-of-each-digit
public int[] NumbersIn(int value) {
var numbers = new Stack<int>();
for(; value > 0; value /= 10)
numbers.Push(value % 10);
return numbers.ToArray();
}
// Call this whenever score is updated
private void UpdateScoreUI() {
int[] arrScore = NumbersIn(scoreCount);
for (int i = 2; i > 0; --i) {
// If the number was say, 11, the list will only be { 1, 1 }
// So we will need to handle the last digit that is out-of-index.
if (arrScore.Length - 1 <= i){
int currNumber = arrScore[i];
scoreSpriteRenderers[i].sprite = scoreSpriteRenderers[currNumber];
} else {
scoreSpriteRenderers[i].sprite = scoreSpriteRenderers[0];
}
}
}
Edit
Yes, this method works with Image
objects as well, since they have a sprite
property, and we are only interacting with that property.