You can use several libraries:
Trimesh
Trimesh
is one of the bests; It can be used on Colab to show:
import trimesh
verts = [[50,50,50], [70,40,50], [50,40,80], [80,70,50]]
faces = [[0,1,2], [2,1,3], [1,0,3]]
mesh = trimesh.Trimesh(vertices=verts, faces=faces)
mesh.show()
# for facet in mesh.facets:
# mesh.visual.face_colors[facet] = trimesh.visual.random_color()
# mesh.split()
# mesh.show()
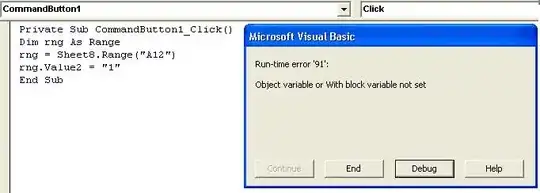
SciPy
1. ConvexHull
:
import scipy as sp
from scipy.spatial import ConvexHull
import matplotlib as mpl
import matplotlib.pyplot as plt
import mpl_toolkits.mplot3d as a3
verts = np.array(verts)
hull = ConvexHull(verts)
indices = hull.simplices
faces = verts[indices]
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.set_xlim([35, 85])
ax.set_ylim([35, 85])
ax.set_zlim([35, 85])
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.set_zlabel('z')
for f in faces:
face = a3.art3d.Poly3DCollection([f])
face.set_color(mpl.colors.rgb2hex(np.random.rand(3)))
face.set_edgecolor('k')
face.set_alpha(0.5)
ax.add_collection3d(face)
plt.show()
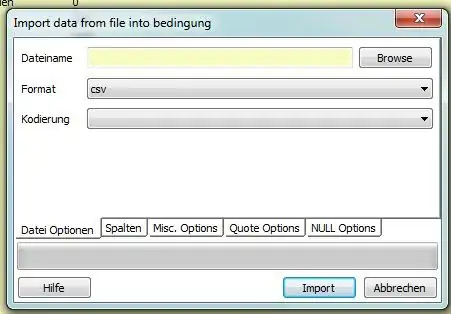
2. Delaunay
:
import numpy as np
import matplotlib.pyplot as plt
from scipy.spatial import Delaunay
verts = np.array(verts)
tri = Delaunay(verts)
fig = plt.figure()
ax = fig.add_subplot(1, 1, 1, projection='3d')
ax.azim = 70
ax.plot_trisurf(verts[:, 0], verts[:, 1], verts[:, 2], triangles=tri.simplices, cmap=plt.cm.Spectral)
plt.show()
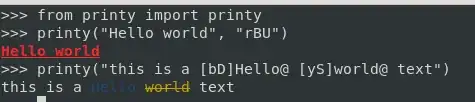