You don't need to detect the home button... in fact, that's probably not what you want to do anyway.
Another approach is to use two bottom constraints, with different priorities.
You can constrain the bottom of the button to the bottom of the safe area, and give that constraint a less-than-required priority.
You can then apply another constraint, this time at a minimum distance from the bottom of the view.
Here's an example:
class BottomPaddingViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let safeAreaView = UIView()
safeAreaView.backgroundColor = .red
safeAreaView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(safeAreaView)
let btn = UIButton()
btn.backgroundColor = .systemBlue
btn.setTitle("Button", for: [])
btn.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(btn)
let g = view.safeAreaLayoutGuide
// bottom constraint for the button
// to the safe area bottom
// with less-than-required priority
let bConstraint = btn.bottomAnchor.constraint(equalTo: g.bottomAnchor)
bConstraint.priority = .required - 1
NSLayoutConstraint.activate([
// constrain the red view to the safe area bottom
safeAreaView.bottomAnchor.constraint(equalTo: g.bottomAnchor),
safeAreaView.leadingAnchor.constraint(equalTo: g.leadingAnchor),
safeAreaView.trailingAnchor.constraint(equalTo: g.trailingAnchor),
safeAreaView.heightAnchor.constraint(equalToConstant: 40.0),
// constrain button 200-pts wide, centered horizontally
btn.widthAnchor.constraint(equalToConstant: 200.0),
btn.heightAnchor.constraint(equalToConstant: 40.0),
btn.centerXAnchor.constraint(equalTo: g.centerXAnchor),
// and bottom AT LEAST 20-points from the bottom of the VIEW
btn.bottomAnchor.constraint(lessThanOrEqualTo: view.bottomAnchor, constant: -20.0),
// activate the button's safe area bottom constraint
bConstraint,
])
}
}
When running on a phone without a physical button (iPhone 12, for example) it looks like this:
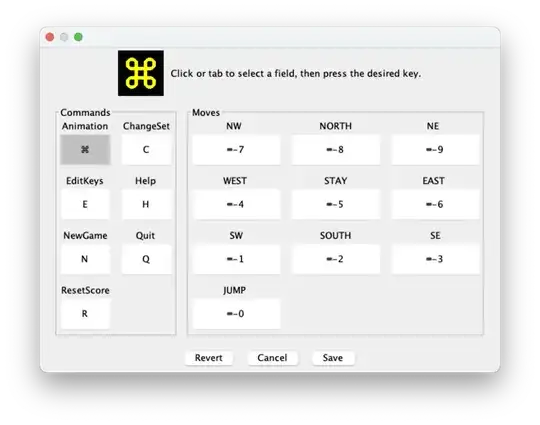
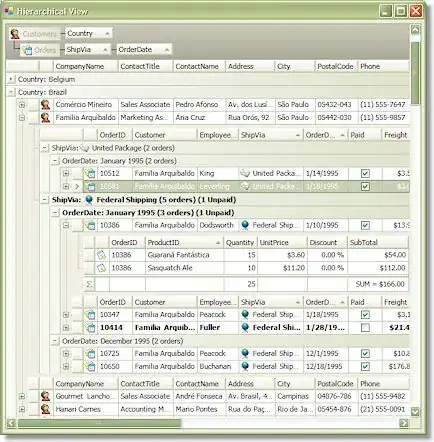
we see that both the blue button and the red view are bottom-constrained to the safe area.
When running on a phone with a physical button (iPhone 8, for example) it looks like this:
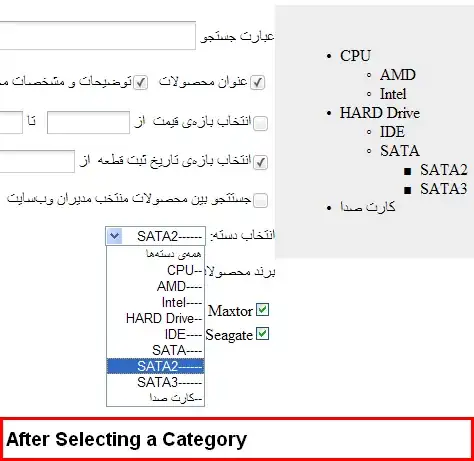
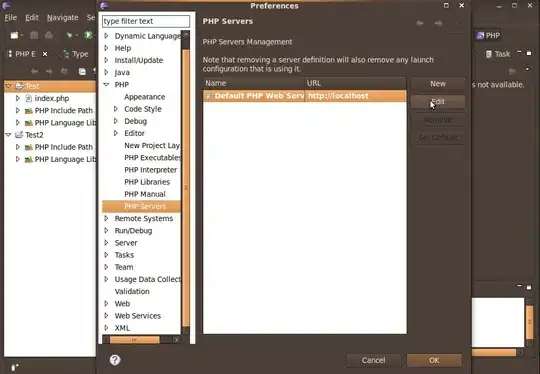
the red view is still constrained to the safe area, but the blue button keeps its minimum distance from the view bottom.