although I'm not a expert in selenium but just try to solve this problem. I create a index.html file to get the options from the dropdown menu. I only create a drop down menu in this index.html files its code is this:-
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div class="row">
<span class="label">Select Text</span>
<span class="box"><input readonly="true" autocapitalize="none" autocomplete="off" autocorrect="off" spellcheck="false" tabindex="0" type="text" aria-autocomplete="list" id="datatext" placeholder="Select an instance" class="datatext" >
<select class="contentselect"
id="contentselect">
<option></option>
<option value="one">test1</option>
<option value="two">test2</option>
<option value="two">test3</option>
<option value="two">test4</option>
<option value="two">test5</option>
<option value="two">test6</option>
</select></span>
</div>
<script>
textfield = document.getElementById("datatext");
contentselect = document.getElementById("contentselect");
contentselect.onchange = function () {
var text = contentselect.options[contentselect.selectedIndex].value
if (text != "") {
textfield.value += text;
}
}
</script>
</body>
</html>
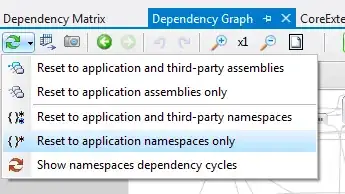
Then I create a java file to read all the options from the index.html. Java code looks like:-
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "D:\\Goal Projects\\Webnovel API\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("http://127.0.0.1:5500/index.html");
Select allOptions = new Select(driver.findElement(By.xpath("//select[@id='contentselect']")));
List<WebElement> elements = allOptions.getOptions();
List<String> options = new ArrayList<String>();
for (WebElement element : elements) {
options.add(element.getText());
}
for (String s : options) System.out.println(s);
}
Result Output is like this:-
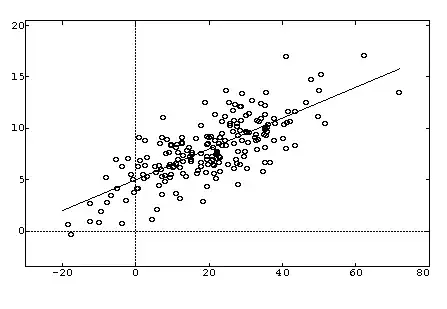
I think you are using wrong xpath you are using input xpath but you should use selection path.
I hope this will help you, Thanks for reading.