If you're just looking to get a string of all the HTML, you can do so via the executeJavaScript
API:
const {app, BrowserWindow, dialog} = require('electron')
async function createWindow () {
const mainWindow = new BrowserWindow()
await mainWindow.loadFile('index.html')
const result = await mainWindow.webContents.executeJavaScript("document.documentElement.outerHTML");
dialog.showMessageBox(mainWindow, {
message: result
});
}
app.whenReady().then(() => {
createWindow()
app.on('activate', function () {
if (BrowserWindow.getAllWindows().length === 0) createWindow()
})
})
app.on('window-all-closed', function () {
if (process.platform !== 'darwin') app.quit()
})
For an HTML page like:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link href="./styles.css" rel="stylesheet">
<title>Hello World!</title>
</head>
<body>
<h1>Hello World!</h1>
We are using Node.js <span id="node-version"></span>,
Chromium <span id="chrome-version"></span>,
and Electron <span id="electron-version"></span>.
<iframe src="https://google.com/chrome"></iframe>
</body>
</html>
You'll get a dialog like this:
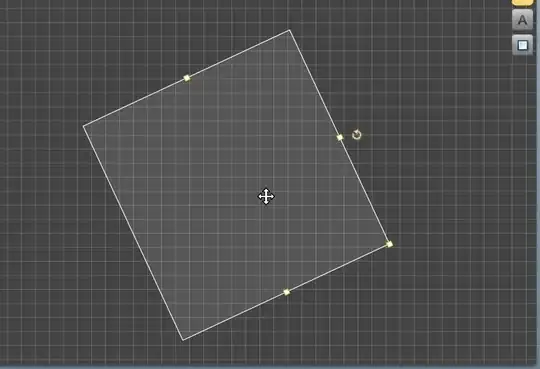
It is not however possible to just grab DOM elements in the main process that you can manipulate.