You can use ax.xaxis.set
to set those properties:
import matplotlib.pyplot as plt
import numpy as np
image = np.random.randint(0, 255, size=(64, 64))
fig, ax = plt.subplots()
im = ax.imshow(image)
cbar = fig.colorbar(im)
ax.xaxis.set(
ticks_position="top",
label_position="top",
label_text="XAxis Label",
)
plt.show()
Resulting plot:
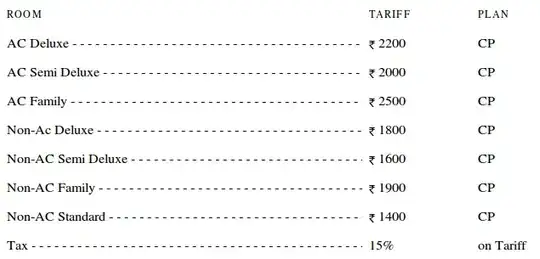
You can see all properties that you can set using ax.xaxis.set?
in IPython / Jupyter Notebook:
>>> ax.xaxis.set?
Signature:
ax.xaxis.set(
*,
agg_filter=<UNSET>,
alpha=<UNSET>,
animated=<UNSET>,
clip_box=<UNSET>,
clip_on=<UNSET>,
clip_path=<UNSET>,
data_interval=<UNSET>,
gid=<UNSET>,
in_layout=<UNSET>,
inverted=<UNSET>,
label=<UNSET>,
label_coords=<UNSET>,
label_position=<UNSET>,
label_text=<UNSET>,
major_formatter=<UNSET>,
major_locator=<UNSET>,
minor_formatter=<UNSET>,
minor_locator=<UNSET>,
path_effects=<UNSET>,
picker=<UNSET>,
pickradius=<UNSET>,
rasterized=<UNSET>,
remove_overlapping_locs=<UNSET>,
sketch_params=<UNSET>,
snap=<UNSET>,
tick_params=<UNSET>,
ticklabels=<UNSET>,
ticks=<UNSET>,
ticks_position=<UNSET>,
transform=<UNSET>,
units=<UNSET>,
url=<UNSET>,
view_interval=<UNSET>,
visible=<UNSET>,
zorder=<UNSET>,
)
Docstring:
Set multiple properties at once.
Supported properties are
Properties:
agg_filter: a filter function, which takes a (m, n, 3) float array and a dpi value, and returns a (m, n, 3) array
alpha: scalar or None
animated: bool
clip_box: `.Bbox`
clip_on: bool
clip_path: Patch or (Path, Transform) or None
data_interval: unknown
figure: `.Figure`
gid: str
in_layout: bool
inverted: unknown
label: object
label_coords: unknown
label_position: {'top', 'bottom'}
label_text: str
major_formatter: `~matplotlib.ticker.Formatter`, ``str``, or function
major_locator: `~matplotlib.ticker.Locator`
minor_formatter: `~matplotlib.ticker.Formatter`, ``str``, or function
minor_locator: `~matplotlib.ticker.Locator`
path_effects: `.AbstractPathEffect`
picker: None or bool or float or callable
pickradius: float
rasterized: bool
remove_overlapping_locs: unknown
sketch_params: (scale: float, length: float, randomness: float)
snap: bool or None
tick_params: unknown
ticklabels: sequence of str or of `.Text`\s
ticks: list of floats
ticks_position: {'top', 'bottom', 'both', 'default', 'none'}
transform: `.Transform`
units: units tag
url: str
view_interval: unknown
visible: bool
zorder: float