I try this Example :
QPushButton *button = new QPushButton();
button->setText("Clicke me ");
ui->gridLayout->addWidget(button);
connect(button,&QPushButton::clicked,this,[](){
qDebug()<<"button is clicked";
}, Qt::UniqueConnection);
what you write In your connect
is wrong, because you should follow this style :
connect(object,SIGNAL(mySig()), this,SLOT(mySlot())),Qt::QueuedConnection);
you can't use two Connections at the same time
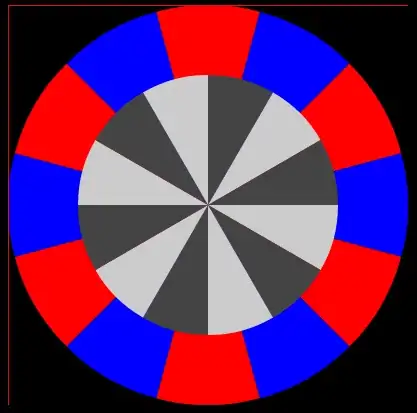
From Qt::ConnectionType for Qt::UniqueConnection
:
This is a flag that can be combined with any one of the above
connection types, using a bitwise OR. When Qt::UniqueConnection is
set, QObject::connect() will fail if the connection already exists
(i.e. if the same signal is already connected to the same slot for the
same pair of objects). This flag was introduced in Qt 4.6.
Noted that it was introduced in Qt 4.6 and it said that if the connection already exists QObject::connect()
will fail.
as I try
connect(button,&QPushButton::clicked,this,[](){
qDebug()<<"button is clicked";
}, Qt::UniqueConnection or Qt::QueuedConnection);
I got an error too because I use the Qt 6
version and it may work in Qt 4.6
.
From https://stackoverflow.com/a/20717599/9484913 I try this :
QPushButton *button = new QPushButton();
button->setText("Clicke me ");
ui->gridLayout->addWidget(button);
QObject::connect(button,&QPushButton::clicked,this,[](){
qDebug()<<"button is clicked";
}, static_cast<Qt::ConnectionType>(Qt::QueuedConnection | Qt::UniqueConnection));
and it works:
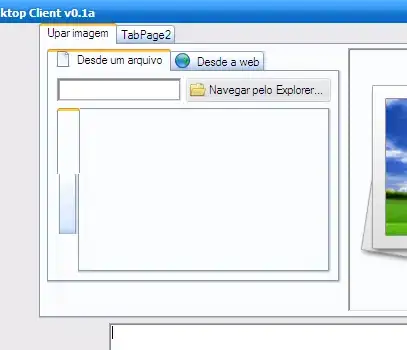