I found a solution for this.
I keep the email field and created a select
add_filter( 'woocommerce_checkout_fields', 'wc_custom_email_phone_field_checkout' );
function wc_custom_email_phone_field_checkout( $fields ) {
$fields['billing']['billing_contact'] = array(
'label' => __('How should we contact you?', 'woocommerce'),
'placeholder' => __('', 'placeholder', 'woocommerce'),
'required' => false,
'clear' => false,
'type' => 'select',
'options' => array(
'email' => __('Email', 'woocommerce' ),
'phone' => __('WhatsApp', 'woocommerce' )
)
);
return $fields;
}
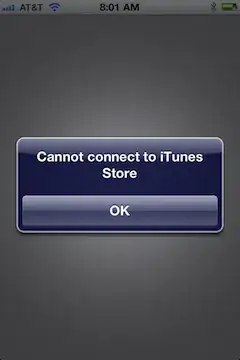
I also created an input
add_filter('woocommerce_checkout_fields', 'custom_woocommerce_whatsapp_fields');
function custom_woocommerce_whatsapp_fields( $fields ){
$fields['billing']['billing_whatsapp'] = array(
'label' => __('Enter your WhatsApp number', 'woocommerce'),
'placeholder' => __('Enter your WhatsApp number', 'woocommerce'),
'required' => false, // if field is required or not
'clear' => false, // add clear or not
'type' => 'text', // add field type
'class' => array('whatsapp'), // add class name
'priority' => 25, // Priority sorting option
);
return $fields;
}
The input field is invisible with css.
.form-row.whatsapp {
display: none;
}
If the option phone is selected, it becomes visible.
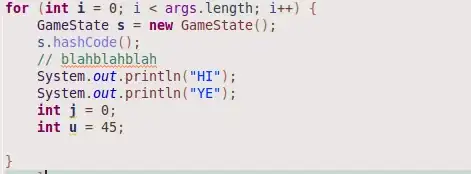
let selectEl = document.getElementById('billing_contact');
selectEl.addEventListener('change', (e) => {
if (e.target.value == 'phone') {
document.getElementById('billing_whatsapp_field').style.display = 'block';
document.getElementById('billing_whatsapp_field').classList.add('validate-required');
} else {
document.getElementById('billing_whatsapp_field').style.display = 'none';
document.getElementById('billing_whatsapp_field').classList.remove('validate-required');
}
});
And finally, if the phone option is selected and form sent with the phone field empty:
add_filter( 'woocommerce_checkout_fields' , 'whatsapp_checkout_fields', 9999 );
function whatsapp_checkout_fields( $fields ) {
if (($_POST['billing_contact'] == 'phone')) {
$fields['billing']['billing_whatsapp']['required'] = true;
} else {
$fields['billing']['billing_whatsapp']['required'] = false;
}
return $fields;
}

If the mail option is selected, the WhatsApp field is not required.
In both cases, I wanted to keep the email as a required field for several reasons. But you can make it false when the phone is selected.