I don't know specifically what you want, as your description is a bit vague (it's always helpful to draw what you want in something like Inkscape, powerpoint or even paint when asking questions about graphs), so here's some possible solutions. First, using ax.fill_between
.
Here's how to add a bar between your two indicated values:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
data=range(20)
ax.plot(data)
ax.plot(2.5,2.5, marker="|",color='blue',markerfacecolor='none',mew=0.5,ms=10,alpha=0.5)
ax.plot(5.0,5.0, marker="|",color='blue',markerfacecolor='none',mew=0.5,ms=10,alpha=0.5)
ax.fill_between([2.5, 5.0], data[0], data[-1], alpha=0.5)
plt.show()
Results in:
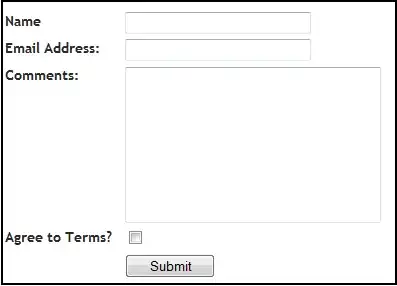
Maybe you want so modify the region a bit, create a smaller square. Modify the fill_between
command to this:
ax.fill_between([2.5, 5.0], 2.5, 5.0, alpha=0.5)
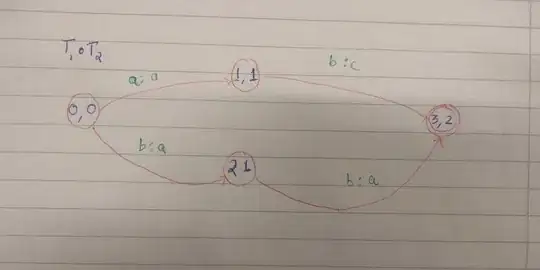
Perhaps you want something more like a "confidence interval". Then you'll need to modify your code a bit. Here's an example
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
data=np.arange(0, 20.5, step=0.5)
ax.plot(data, data)
ax.plot(2.5,2.5, marker="|",color='blue',markerfacecolor='none',mew=0.5,ms=10,alpha=0.5)
ax.plot(5.0,5.0, marker="|",color='blue',markerfacecolor='none',mew=0.5,ms=10,alpha=0.5)
ax.fill_between(data, data-1, data+1, alpha=0.5, where=(data >= 2.5) & (data <= 5.0))
plt.show()
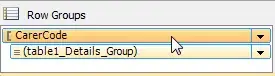
EDIT: Now I saw you mentioned axvspan
. It's very similar to fill_between
. If you replace fill_between
in the first example with this:
ax.axvspan(2.5, 5.0, ymin=0, ymax=17, alpha=0.5)
You get this:
