We have two options to resolve the problem with fullscreen mode.
First solution:
In styled-components you will need to use the @media all and (display-mode: fullscreen)
instead of the :fullscreen
pseudo-class, because this pseudo-class works only with Fullscreen API.
This will triggered with F11 key as an on/off switch, however we cann't use the Esc key to cancel the fullscreen mode.
const NavbarContainer = styled.div`
height: 30px;
background-color: mediumseagreen;
padding: 10px 20px;
display: flex;
justify-content: space-between;
align-items: center;
color: white;
@media all and (display-mode: fullscreen) {
display: none;
}
`;
Second solution:
We using Fullscreen API, when we will call this API after that the :fullscreen
pseudo-class will works. This approach is good if you want to use Esc key to exit from fullscreen mode, also to assign another key (like Enter) or element (like button) to trigger fullscreen mode.
The Fullscreen API adds methods to present a specific Element (and its descendants) in fullscreen mode, and to exit fullscreen mode once it is no longer needed. This makes it possible to present desired content—such as an online game—using the user's entire screen, removing all browser user interface elements and other applications from the screen until fullscreen mode is shut off. MDN
Although, if we using @media
query in our css instead of :fullscreen
pseudo-class, we also can to use F11 key as a trigger. As a plus, with this approach, we will recive two different notifications about of exiting the fullscreen mode.
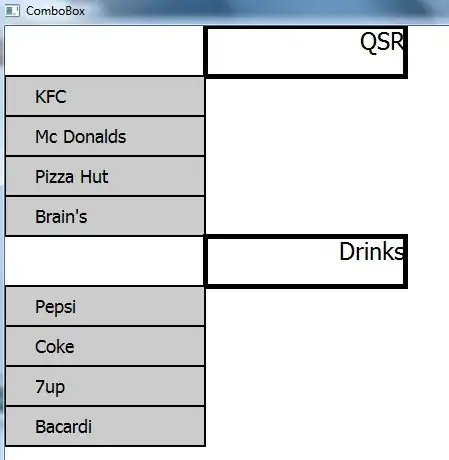
import { useEffect, useRef } from "react";
import styled from "styled-components";
const NavbarContainer = styled.div`
height: 30px;
background-color: mediumseagreen;
padding: 10px 20px;
display: flex;
justify-content: space-between;
align-items: center;
color: white;
@media all and (display-mode: fullscreen) {
display: none;
}
`;
const FullScreenButton = styled.button`
padding: 0.5rem 1rem;
@media all and (display-mode: fullscreen) {
display: none;
}
`;
export default function App() {
const refNavbar = useRef<HTMLDivElement>(null);
useEffect(() => {
const navbar = refNavbar.current;
const actionFn = (event: KeyboardEvent) => {
if ((event.key === 'Enter') && navbar) {
navbar.requestFullscreen();
return;
}
};
document.addEventListener('keyup', actionFn, false);
return () => {
document.removeEventListener('keyup', actionFn, false);
};
}, []);
const fullScreenOnClick = () => {
const navbar = refNavbar.current;
if (!navbar) return;
navbar.requestFullscreen();
};
return (
<div className="App">
<NavbarContainer ref={refNavbar}>Navigation</NavbarContainer>
<section>
<p>(User content!)</p>
<FullScreenButton onClick={fullScreenOnClick}>Fullscreen mode</FullScreenButton>
</section>
</div>
);
}

Caveat: Fullscreen API with assigned F11 key as trigger.
If you will try to assign the F11 key using the Fullscreen API to be able to exit also with the Esc key you will get strange behavior. It may be the cause of two different events Fullscreen API (with Esc key) and F11 key.
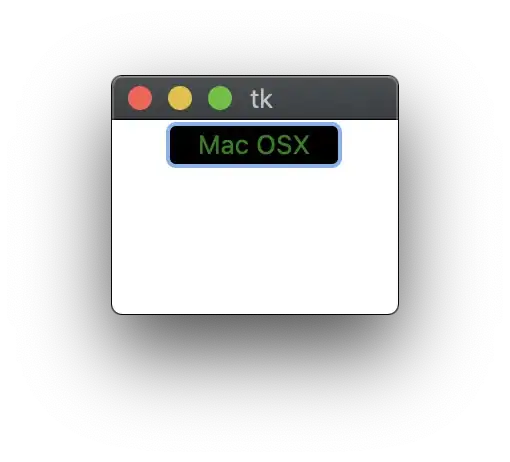
Fullscreen API issue with F11 and Esc buttons.
F11 key can exit programmatic-fullscreen, but programmatic-exitFullscreen cannot exit F11-fullscreen. Which is the problem you are running into. Also, Esc key cannot exit F11-fullscreen, but does exit programmatic-fullscreen. Fullscreen API not working if triggered with F11
Additionally:
Another interesting issue about :fullscreen
pseudo-class. If you want to hide more than one element (like a button), this doesn't works as it will be bind to the current element. It's better to use the @media query.
Navbar and botton have a :fullscreen
pseudo-class
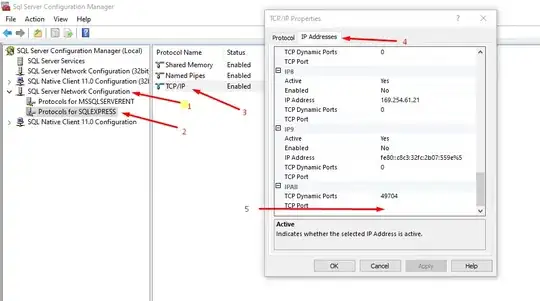