You could extract the contents of your database as a flat table like this, then cycle over the records to build a graph
in a module like NetworkX
In the below, I setup a pandas dataframe to host the data, but for you, you'd need to deal with whatever database api you've chosen:
import pandas as pd
import networkx as nx
df = pd.DataFrame([{"Mother" : '0x123',
"Child1" : '0x456',
"Child2" : '0x789',
"Salary" : 80},
{"Mother" : '0x456',
"Child1" : '0xabc',
"Child2" : None,
"Salary" : 60},
{"Mother" : '0x789',
"Child1" : None,
"Child2" : None,
"Salary" : 50},
{"Mother" : '0xabc',
"Child1" : '0xdef',
"Child2" : '0xghi',
"Salary" : 120},
],
)
graph = nx.DiGraph()
for e, r in df.iterrows():
graph.add_node(r.Mother, data={"salary" : r.Salary})
if r.Child1 is not None:
graph.add_edge(r.Mother, r.Child1)
if r.Child2 is not None:
graph.add_edge(r.Mother, r.Child2)
This will instantiate your graph, for the above data, the graph looks like this:
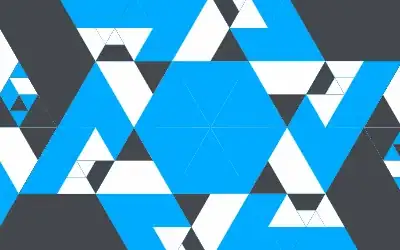
Once instantiated, you can then extract details such as all the descendants of a given node.
e.g.
summary=[]
start_node = '0x456'
node_set = nx.descendants(graph, start_node)
node_set.add(start_node)
for node,data in graph.nodes(data=True):
print(data)
if node in node_set:
summary.append(data.get('data',{}).get('salary',0))
Which you can finally use to calculate your tree-based figure:
summary
Which returns:
>>> [60, 120, 0, 0]
So summing this:
sum(summary)
Finally returns:
>>> 180