UPDATED**
Updated example code.
Data from each sheet in each workbook in your path is pasted down the default sheet in the created combined workbook. Each workbook contents is separated by 'row_separation' rows.
Each sheet in the workbook is pasted across the combined sheet starting at Column 'A' and separated by 'col_separation' columns.
You'd need to determine where each original sheet gets pasted into Sheet1 in the combined excel file.
Note; this copy/paste should also include the data styling like font name, color, size and format.
row_separation = 100
col_separation = 20
row = 1
with xw.App(visible=False) as app:
combined_wb = app.books.add()
for excel_file in excel_files:
col = 1
print("Reading Excel file: " + excel_file)
wb = app.books.open(excel_file)
for sheet in wb.sheets:
ws = wb.sheets[sheet]
wb_name = str(wb.name)
sheet_name = str(sheet.name)
print("Extracting data from " + wb_name + "-" + sheet_name)
combined_wb.sheets[0].range(row, col).value = 'Data from Workbook: ' + wb_name + ' Sheet: ' + sheet_name
combined_wb.sheets[0].range(row, col).font.bold = True
ws.used_range.copy()
combined_wb.sheets[0].range(row+1, col).paste(paste='all')
col += col_separation
wb.close()
row += row_separation
combined_wb.save(outputFile)
combined_wb.close()
Example sheet from 3 workbooks, workbook1 has 2 sheets, workbook2 has 3 sheets and workbook3 has 1 sheet. Separation is set to 10 for rows and 8 for columns for display.
Answer 2 ----------------------------------
Selecting the same worksheets from the same workbooks and adding to combined_wb as separate sheets.
with xw.App(visible=False) as app:
combined_wb = app.books.add()
first_sheet = combined_wb.sheets[0]
for excel_file in excel_files:
col = 1
print("Reading Excel file: " + excel_file)
wb = app.books.open(excel_file)
for sheet in wb.sheets:
ws = wb.sheets[sheet]
wb_name = str(wb.name)
sheet_name = str(sheet.name)
print("Extracting Sheet from " + wb_name + "-" + sheet_name)
ws.api.Copy(After=first_sheet.api)
first_sheet = combined_wb.sheets[sheet_name]
wb.close()
# combined_wb.sheets[0].delete() # Delete initial Sheet1 if not required
combined_wb.save(outputFile)
combined_wb.close()
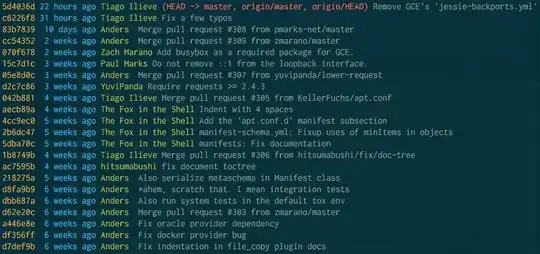