Note: I assume you're not looking for all emoji, since they include quite common characters:
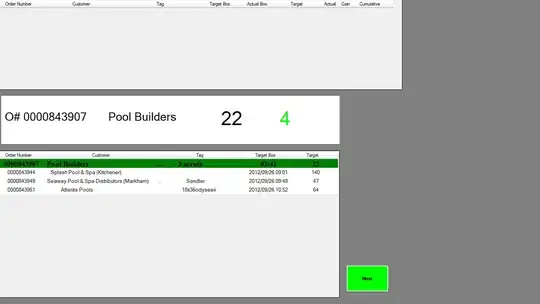
(from https://unicode.org/Public/UNIDATA/emoji/emoji-data.txt)
Unicode library
To get the Unicode block for one or more characters, we can use the Unicode library:
library("Unicode") # install.packages("Unicode")
A few examples:
> "" |> utf8ToInt() |> u_char_properties("Block")
Block
U+1F60E Emoticons
> "" |> utf8ToInt() |> u_char_properties("Block")
Block
1 Miscellaneous Symbols and Pictographs
2 General Punctuation
3 Miscellaneous Symbols and Pictographs
4 General Punctuation
5 Miscellaneous Symbols and Pictographs
> "" |> utf8ToInt() |> u_char_properties("Block")
Block
U+1F910 Supplemental Symbols and Pictographs
> "✅" |> utf8ToInt() |> u_char_properties("Block")
Block
U+2705 Dingbats
> "☝️" |> utf8ToInt() |> u_char_properties("Block")
Block
U+261D Miscellaneous Symbols
U+FE0F Variation Selectors
> "☎️" |> utf8ToInt() |> u_char_properties("Block")
Block
U+260E Miscellaneous Symbols
U+FE0F Variation Selectors
> "♍" |> utf8ToInt() |> u_char_properties("Block")
Block
U+264D Miscellaneous Symbols
> "" |> utf8ToInt() |> u_char_properties("Block")
Block
U+1FAC3 Symbols and Pictographs Extended-A
U+1F3FD Miscellaneous Symbols and Pictographs
> "" |> utf8ToInt() |> u_char_properties("Block")
Block
U+1F682 Transport and Map Symbols
Matching all emoji-like characters could be done like this:
blocks <- c("Emoticons",
"Miscellaneous Symbols and Pictographs",
"Supplemental Symbols and Pictographs",
"Dingbats",
"Miscellaneous Symbols",
"Symbols and Pictographs Extended-A",
"Transport and Map Symbols")
> "" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "☎️" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "♍" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] TRUE
> "#" |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0
[1] FALSE
Integrating into your code
library(rtweet)
library(dplyr)
get_token() # Connects with Twitter API
Uber <- search_tweets("uber", n = 100, lang = "en")
Uber_filtered <- Uber %>%
rowwise() %>%
filter(text |> utf8ToInt() |> u_char_properties("Block") |> unlist() |> intersect(blocks) |> length() > 0)