So, this was an absolute headache but I finally figured out a satisfactory way to access and use these variables in code.
My solution uses:
- A (gitignored) JSON file to store the variables locally
- Xcode Cloud to send the variables in the CI
- A
ci_pre_xcodebuild.sh
file to write the environment variables in the JSON
- A Swift file that allows you to conveniently access the secrets.
Step 1: Basic JSON file
- In your .gitignore file, add a new entry for the JSON file and its path
- Create a JSON file through Xcode
- Add your keys to this JSON file.
Secrets.json
(at: YourProject/SupportingFiles/secrets.json)
{
"STRIPE_KEY": "",
"GOOGLE_MAPS_KEY": "",
"GOOGLE_PLACES_KEY": "",
"BASE_URL": "https://dev.api.example.fr"
}
Step 2: Write the variables in Xcode Cloud
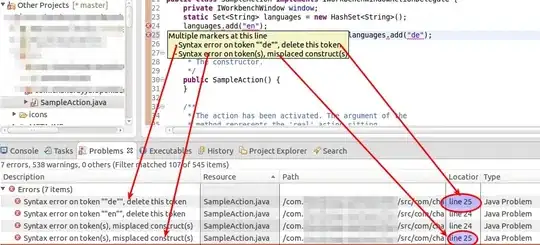
In this screenshot you can see that I've duplicated the keys for different environments. I didn't expand on this for the sake of brevity, but you can definitely have different secrets JSON files for different Xcode Scheme configurations.
Step 3: Add a ci_pre_xcodebuild.sh
file
Important: the name of the files and their position matter.
The goal here is to add a script that the CI (Xcode Cloud) will execute each time it builds. In this script, we're going to create and fill our JSON.
- Add a new group at the root of your project named "ci_scripts"
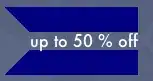
In this group, add a new file called ci_pre_xcodebuild.sh
Write the following:
#!/bin/sh
echo "Stage: PRE-Xcode Build is activated .... "
# Move to the place where the scripts are located.
# This is important because the position of the subsequently mentioned files depend of this origin.
cd $CI_WORKSPACE/ci_scripts || exit 1
# Write a JSON File containing all the environment variables and secrets.
printf "{\"STRIPE_KEY\":\"%s\",\"GOOGLE_MAPS_KEY\":\"%s\",\"GOOGLE_PLACES_KEY\":\"%s\",\"BASE_URL\":\"%s\"}" "$STRIPE_KEY" "$GOOGLE_MAPS_KEY" "$GOOGLE_PLACES_KEY" "$BASE_URL" >> ../Dream\ Energy/SupportingFiles/Secrets.json
echo "Wrote Secrets.json file."
echo "Stage: PRE-Xcode Build is DONE .... "
exit 0
Of course, you need to change this text depending on your keys and the location of the file. I added a few keys as an example.
- In your Terminal, navigate to the new file's folder and run this command:
chmod +x ci_pre_xcodebuild.sh
. This fixes a warning in Xcode Cloud.
Step 4: [BONUS] A simple Swift file to access the environment variables
import Foundation
struct Secrets {
private static func secrets() -> [String: Any] {
let fileName = "Secrets"
let path = Bundle.main.path(forResource: fileName, ofType: "json")!
let data = try! Data(contentsOf: URL(fileURLWithPath: path), options: .mappedIfSafe)
return try! JSONSerialization.jsonObject(with: data) as! [String: Any]
}
static var baseURL: String {
return secrets()["BASE_URL"] as! String
}
static var stripeKey: String {
return secrets()["STRIPE_KEY"] as! String
}
}