Problem:
I don't believe this is possible with rvest currently as it relies on selectors level 3 under the hood, which only permits a simple selector with negation i.e. inside of :not().
The rows are all at the same DOM level and what you want is to be able to filter out later rows from the first "batch".
What would work, with selectors level 4 which permits selector lists inside of :not(), is:
tr[style]:nth-child(1) ~ tr:not(tr[style]:nth-child(n+2) ~ tr):not([style]) td:first-child
or
tr[style]:nth-child(1) ~ tr:not([style], tr[style]:nth-child(n+2) ~ tr) td:first-child
The above are just working examples for selectors level 4. There are other variants. In the above, a complex selector list is passed to :not() to filter out the next result and any subsequent sibling rows.
See selectors by level here.
I think this limitation is a deliberate consequence of the implementation detail of selectr under the hood. Within the source code, if :not
is encountered, the next delimiter is expected to be ")". Rejected are elements that would comprise a selector list such as operators e.g. ~, +. In the current source code you can view this detail on lines 510-528.
Compare the following:
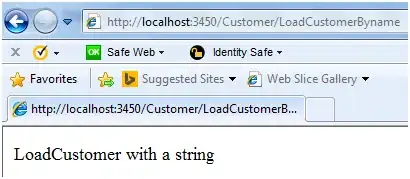
parse_simple_selector, which is a selectr function, objects to the presence of a non-simple selector inside the pseudo class negation.
library(rvest)
link <- 'https://clinicaltrials.gov/ct2/results/details?cond=breast+cancer'
page <- read_html(link)
selector_list <- 'tr[style]:nth-child(1) ~ tr:not(tr[style]:nth-child(n+2) ~ tr):not([style]) td:first-child'
page |> html_elements(selector_list) # fail
page |> html_element('tr:not(td ~ td)') # fail
page |> html_element('tr:not(td)') # pass
Now, look at soupsieve, a python package used by Beautiful Soup 4, which:
provides selectors from the CSS level 1 specifications up through the
latest CSS level 4 drafts and beyond (though some are not yet
implemented).
as at 28/7/22
The implemented details from selectors level 4 allow for complex selector lists within :not()

import requests
from bs4 import BeautifulSoup as bs
selector = 'tr[style]:nth-child(1) ~ tr:not( tr[style]:nth-child(n+2) ~ tr):not([style]) td:first-child'
soup = bs(requests.get('https://clinicaltrials.gov/ct2/results/details?cond=breast+cancer').text, 'html.parser')
soup.select(selector)
Solution:
Some possible options might include:
- Implement your own helper(s) that extend selectr
- Utilise a loop that stops when it hits the next tr with a style attribute following finding the first with your target text
- Preferred solution, IMO, switch to xpath e.g.
(//tr[count(preceding-sibling::tr[@style])=1 and count(following-sibling::tr[@style])>=2])//td[1]
In code
page |> html_elements(xpath = '(//tr[count(preceding-sibling::tr[@style])=1 and count(following-sibling::tr[@style])>=2])//td[1]') |> html_text(trim = T)
Now, these are positional matchings so you might decide to improve by using some text based matching involving your search term.
The idea of using count within xpath I got from @Daniel Haley here