If you want to avoid VBA, you could just do a formula...put the below in cell K2
(or wherever...) and drag down.
=TRIM(IF(J2="Company One",SUBSTITUTE(H2,IFERROR(MID(H2,FIND(".",H2)-1,2),""),""),H2))
You could also have spill range as shown in column L
in screen shot.
=FILTER(TRIM(IF(J2:J9999="Company One",SUBSTITUTE(H2:H9999,IFERROR(MID(H2:H9999,FIND(".",H2:H9999)-1,2),""),""),H2:H9999)),H2:H9999<>"")
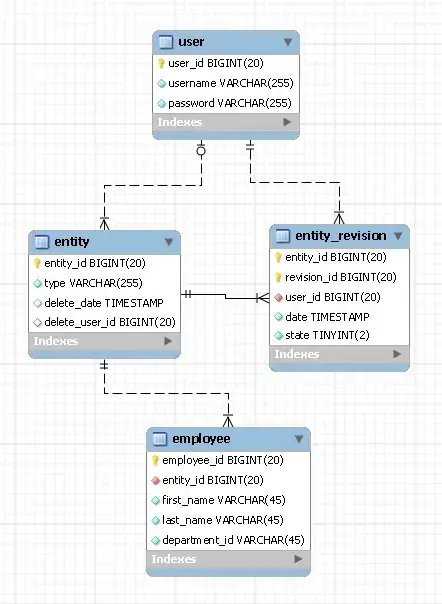
If you really want a macro, here's a dynamic one that is pretty straight forward. A perfect answer would do an array, but looping through helps see what's happening.
Sub doReplace()
Dim changeRange As Range, aCell As Range, aPosition As Long
Const companySkip = "Company One"
Set changeRange = Intersect(Range("H:H"), ActiveSheet.UsedRange).Offset(1, 0)
For Each aCell In changeRange.Cells
If aCell.Offset(0, 2).Value = companySkip Then
aPosition = InStr(1, aCell.Value, ".", vbBinaryCompare)
If aPosition > 0 Then
aCell.Value = Trim(Replace(aCell.Value, Mid(aCell.Value, aPosition - 1, 2), ""))
End If
End If
Next aCell
End Sub