I have a splash screen that I show when the app launch. I'm using SplashScreenAPI for the splash screen. I'm also using accompanist navigation library to navigate with animations. After I updated the accompanist version from 0.24.7-alpha to 0.24.8-beta I've encountered an issue. The issue is :
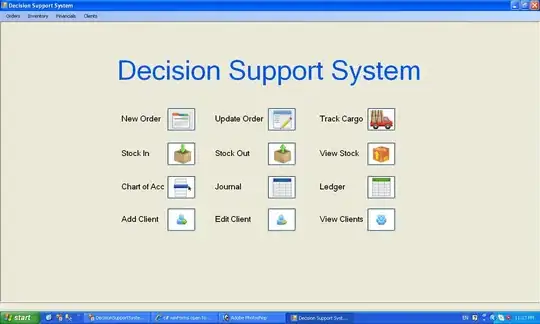
As you can see after the splash screen is shown there is a blank screen for a sec, then it navigates to the start destination.
Here is also the behavior for version 0.24.7-alpha:
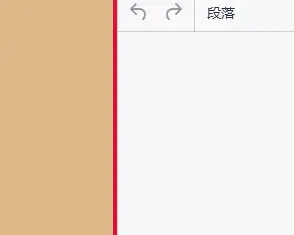
In case of need here is also the code:
@OptIn(ExperimentalMaterial3WindowSizeClassApi::class)
@AndroidEntryPoint
class AuthActivity : ComponentActivity() {
private val splashViewModel: SplashViewModel by viewModels()
private lateinit var splashScreen: SplashScreen
override fun onCreate(savedInstanceState: Bundle?) {
splashScreen = installSplashScreen()
super.onCreate(savedInstanceState)
splashScreen.setKeepOnScreenCondition {
splashViewModel.isLoading.value
}
splashScreen.setOnExitAnimationListener {
val startDestination = splashViewModel.navDestination.value.route
if (startDestination == AUTH_GRAPH) {
it.remove()
} else {
val intent = Intent(this@AuthActivity, MainActivity::class.java)
startActivity(intent)
finish()
}
}
setContent {
val errorMessage by splashViewModel.errorFlow.collectAsState()
val widthSizeClass = calculateWindowSizeClass(this).widthSizeClass
ProvideLocalWindowWidthSizeClass(widthSizeClass = widthSizeClass) {
RubiBrandsTheme {
RubiBrandsBackground {
errorMessage?.asString(this@AuthActivity)?.let {
ErrorDialog(message = it)
}
AuthNavGraph(
navController = rememberAnimatedNavController(),
activity = this@AuthActivity
)
}
}
}
}
}
}
I just check the authentication and then navigate the user based on success or not.
Here is my MainActivity
:
@[AndroidEntryPoint OptIn(ExperimentalMaterial3WindowSizeClassApi::class)]
class MainActivity : ComponentActivity(), AuthenticationManager {
private val mViewModel by viewModels<MainActivityViewModel>()
@Inject
lateinit var authenticationMediator: AuthenticationMediator
private lateinit var navController: NavHostController
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
WindowCompat.setDecorFitsSystemWindows(window, false)
authenticationMediator.registerAuthenticationManager(this)
setContent {
val widthSizeClass = calculateWindowSizeClass(this).widthSizeClass
val showUnauthorizedDialog = mViewModel.showUnauthorizedDialog
ProvideLocalWindowWidthSizeClass(widthSizeClass = widthSizeClass) {
RubiBrandsTheme {
RubiBrandsBackground {
navController = rememberAnimatedNavController()
val bottomSheetNavigator =
rememberBottomSheetNavigator()
val scaffoldState = rememberScaffoldState()
navController.navigatorProvider += bottomSheetNavigator
val navBackStackEntry by navController.currentBackStackEntryAsState()
val currentDestination = navBackStackEntry?.destination
val rubiBrandsTopLevelNavigation = remember(navController) {
RubiBrandsTopLevelNavigation(navController)
}
val topLevelDestinations = listOf(
TopLevelDestination.DashboardDestination,
TopLevelDestination.OrdersDestination,
TopLevelDestination.ProductsDestination,
TopLevelDestination.AccountDestination
)
val topLevelDestinationRoutes =
topLevelDestinations.map(TopLevelDestination::route)
val isNavBarVisible =
navBackStackEntry?.destination?.route in topLevelDestinationRoutes
ModalBottomSheetLayout(
modifier = Modifier
.systemBarsPadding()
.imePadding(),
bottomSheetNavigator = bottomSheetNavigator,
sheetElevation = dimensionResource(id = R.dimen.dimen_16),
sheetShape = RoundedCornerShape(
topStart = dimensionResource(id = R.dimen.dimen_16),
topEnd = dimensionResource(
id = R.dimen.dimen_16
)
),
sheetBackgroundColor = RubiBrandsTheme.colors.filterBottomSheetBackgroundColor
) {
RubiBrandsScaffold(
modifier = Modifier
.systemBarsPadding()
.imePadding(),
bottomBar = {
if (isNavBarVisible) {
RubiBrandsBottomNavigationView(
onNavigateToTopLevelDestination = rubiBrandsTopLevelNavigation::navigateTo,
currentDestination = currentDestination,
topLevelDestinations = topLevelDestinations
)
}
},
scaffoldState = scaffoldState
) {
//navigation graph
Box(
modifier = Modifier
.padding(it)
) {
MainNavGraph(
navController = navController,
mainActivity = this@MainActivity,
mainActivityViewModel = mViewModel,
)
showUnauthorizedDialog?.getContentIfNotHandled()?.let { show ->
if (show) {
RubiBrandsUnauthorizedScreen {
logClickEvent(ItemNames.UNAUTHORIZED_DIALOG_OKAY_BUTTON)
logout()
}
}
}
}
}
}
}
}
}
}
}
@Composable
fun MainNavGraph(
navController: NavHostController,
modifier: Modifier = Modifier,
) {
AnimatedNavHost(
navController = navController,
startDestination = MAIN_GRAPH,
) {
//main nav graph
mainNavGraph(navController)
}
fun NavGraphBuilder.mainNavGraph(
navController: NavController
) {
navigation(
startDestination = TopLevelDestination.DashboardDestination.route,
route = MAIN_GRAPH,
) {
addDashboardScreen(navController)
}
}
Here is also compose dependencies and the versions :
object Compose : Library {
object Version {
const val COMPOSE_VERSION = "1.2.0"
const val COMPOSE_ACTIVITY_VERSION = "1.5.1"
const val COMPOSE_CONSTRAINT_LAYOUT_VERSION = "1.0.1"
const val HILT_NAVIGATION_COMPOSE_VERSION = "1.0.0"
}
const val COMPOSE_UI = "androidx.compose.ui:ui:$COMPOSE_VERSION"
const val COMPOSE_MATERIAL = "androidx.compose.material:material:$COMPOSE_VERSION"
const val COMPOSE_UI_TOOLING_PREV =
"androidx.compose.ui:ui-tooling-preview:$COMPOSE_VERSION"
const val COMPOSE_ACTIVITY = "androidx.activity:activity-compose:$COMPOSE_ACTIVITY_VERSION"
const val COMPOSE_UI_TOOLING = "androidx.compose.ui:ui-tooling:$COMPOSE_VERSION"
const val COMPOSE_UI_TEST_MANIFEST = "androidx.compose.ui:ui-test-manifest:$COMPOSE_VERSION"
const val COMPOSE_CONSTRAINT_LAYOUT =
"androidx.constraintlayout:constraintlayout-compose:$COMPOSE_CONSTRAINT_LAYOUT_VERSION"
const val HILT_NAVIGATION_COMPOSE =
"androidx.hilt:hilt-navigation-compose:$HILT_NAVIGATION_COMPOSE_VERSION"
const val COMPOSE_LIVE_DATA = "androidx.compose.runtime:runtime-livedata:$COMPOSE_VERSION"
override val components: List<String>
get() = listOf(
COMPOSE_UI,
COMPOSE_MATERIAL,
COMPOSE_UI_TOOLING_PREV,
COMPOSE_ACTIVITY,
)
}
I know that my compose version should be compatible with the accompanist version. As per this I should set the accompanist version to 0.25.1 but because of this issue, I don't update the animation version.