This example should illustrate the point:
import pandas as pd
import numpy as np
#for_map = pd.read_csv('campaign_contributions_for_map.tsv', sep='\t')
df_airports = pd.read_csv('C:\\airports.csv')
print(df_airports.head(3))
df_cities = pd.read_csv('C:\\worldcities.csv')
print(df_cities.head(3))
# join the two dataframes - must be the same length
df = pd.concat([df_cities, df_airports], axis=1)
# cast latitudes and longitudes to numeric
cols = ["lat", "lng", "latitude_deg", "longitude_deg"]
df[cols] = df[cols].apply(pd.to_numeric, errors='coerce', axis=1)
# create a mask where our conditions are met (difference between lat fuze and lat air < 5 and difference between long fuze and long air < 0.1)
mask = ((abs(df["lat"] - df["latitude_deg"]) < .5) & (abs(df["lng"] - df["longitude_deg"]) < .5))
# fill the type column
df.loc[mask, 'Type'] = "Airport"
df.shape
pd.set_option('display.max_columns', None)
pd.set_option('display.max_rows', None)
df.head()
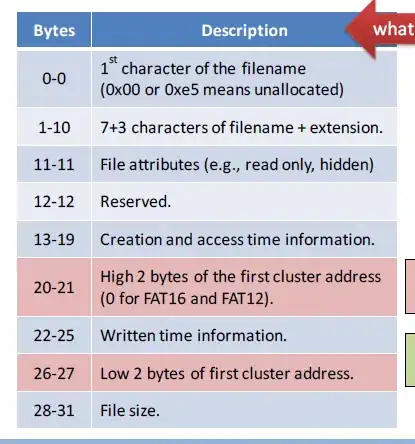
# The haversine formula determines the great-circle distance between two points on a sphere given
# their longitudes and latitudes. Important in navigation, it is a special case of a more general
# formula in spherical trigonometry, the law of haversines, that relates the sides and angles of
# spherical triangles.
lat1 = df['lat']
lon1 = df['lng']
lat2 = df['latitude_deg']
lon2 = df['longitude_deg']
from math import radians, cos, sin, asin, sqrt
def haversine(lat1, lon1, lat2, lon2):
"""
Calculate the great circle distance between two points
on the earth (specified in decimal degrees)
"""
# convert decimal degrees to radians
lon1, lat1, lon2, lat2 = map(radians, [lon1, lat1, lon2, lat2])
# haversine formula
dlon = lon2 - lon1
dlat = lat2 - lat1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * asin(sqrt(a))
# Radius of earth in kilometers is 6371
km = 6371* c
return km
# Creating a new column to generate the output by passing lat long information to Haversine Equation
df['distance'] = [haversine(df.lat[i],df.lng[i],df.latitude_deg[i],df.longitude_deg[i]) for i in range(len(df))]
df['distance'] = df['distance'].round(decimals=3)
# Printing the data table
df.sort_values(by=['distance'], inplace=True)
df.head()
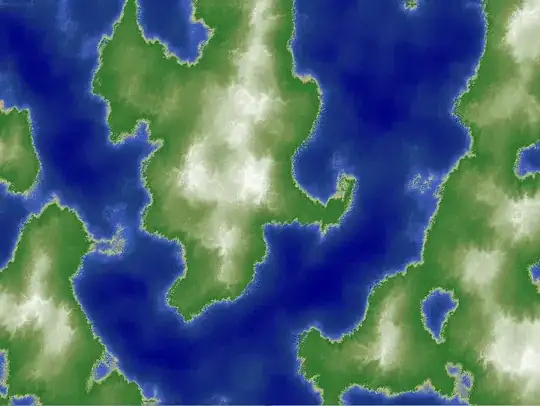
# Let's sort by our newly created field, which identifies airport lat/lonn coordinates within .5 places of
# a city's lat/long coordinates
# Create a mask where our conditions are met (difference between lat and latitude_deg < 0.1 and
# difference between lng and longitude_deg < 0.1)
mask = ((abs(df["lat"] - df["latitude_deg"]) < 0.1) & (abs(df["lng"] - df["longitude_deg"]) < 0.1))
# Fill the type column
df.loc[mask, 'Type'] = "Airport"
df.sort_values(by=['Type'], inplace=True)
df.head()
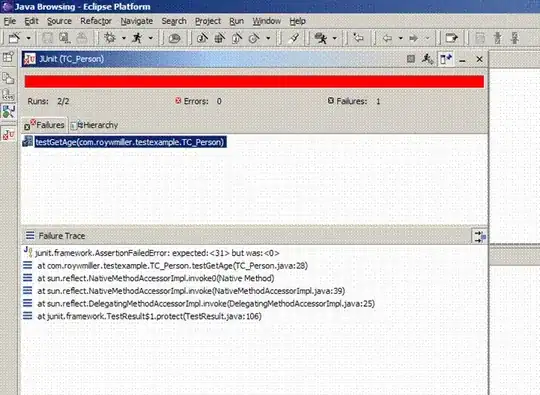
More details here.
https://github.com/ASH-WICUS/Notebooks/blob/master/Haversine%20Distance%20-%20Airport%20or%20Not.ipynb