Your code shows iterating a collection of type Object
and we know for sure that class Object
does have a GetType()
method and _doesn't
_ have a GetName()
method or Name
property. But the item
in your collection most likely isn't "really" an object
, it's some class that (like every class) inherits Object
. You seem confident that this unknown class has a Name
property but even if the object type of item
is completely unknown, you can use System.Reflection
to print out the Name if it exists and an error readout if it doesn't.
Wrapping this reflection in an Extension Method is of the easier ways to answer your question:
[...]How can I create a function to do this where it says GetName()
static partial class Extensions
{
public static string GetName(this object unk)
{
Type type = unk.GetType();
PropertyInfo propertyInfo = type.GetProperty("Name");
if (propertyInfo == null)
{
return $"Error: Type '{type.Name}' does not have a Name property.";
}
else
{
return $"{propertyInfo.GetValue(unk)}";
}
}
}
MOCK
With the extension in place, you can now use the syntax shown in your post.
var collection = new object []
{
new HasNameA(),
new HasNameB(),
new NoNameC(),
};
foreach (object item in collection)
{
Console.WriteLine("variable name : " + item.GetName());
Console.WriteLine("variable value: " + item);
Console.WriteLine("variable type : " + item.GetType().Name);
Console.WriteLine("***************************** ");
}
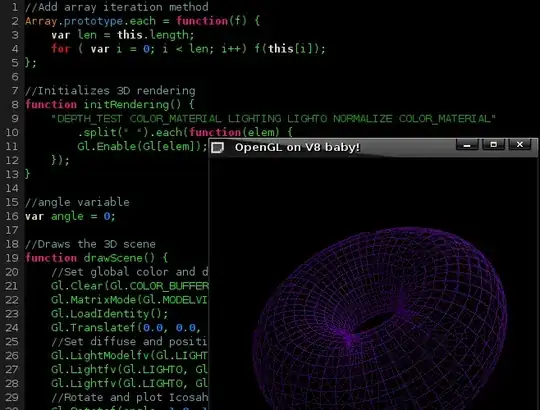
Where:
class HasNameA
{
public string Name { get; set; } = "A";
public object Value { get; set; } = 1;
public override string ToString() => $"{Value}";
}
class HasNameB
{
public string Name { get; set; } = "B";
public object Value { get; set; } = "Hello";
public override string ToString() => $"{Value}";
}
class NoNameC
{
public object Value { get; set; } = Math.PI;
public override string ToString() => $"{Value}";
}