This can be done by writing a custom gesture Modifier such as
fun Modifier.timedClick(
timeInMillis: Long,
interactionSource: MutableInteractionSource = remember {MutableInteractionSource()},
onClick: (Boolean) -> Unit
) = composed {
var timeOfTouch = -1L
LaunchedEffect(key1 = timeInMillis, key2 = interactionSource) {
interactionSource.interactions
.onEach { interaction: Interaction ->
when (interaction) {
is PressInteraction.Press -> {
timeOfTouch = System.currentTimeMillis()
}
is PressInteraction.Release -> {
val currentTime = System.currentTimeMillis()
onClick(currentTime - timeOfTouch > timeInMillis)
}
is PressInteraction.Cancel -> {
onClick(false)
}
}
}
.launchIn(this)
}
Modifier.clickable(
interactionSource = interactionSource,
indication = rememberRipple(),
onClick = {}
)
}
Usage
val context = LocalContext.current
Card(
shape = RoundedCornerShape(percent = 50),
) {
Box(
modifier = Modifier
.timedClick(
timeInMillis = 1000,
) { passed: Boolean ->
Toast
.makeText(
context,
"Pressed longer than 1000 $passed",
Toast.LENGTH_SHORT
)
.show()
}
.fillMaxWidth()
.height(100.dp),
contentAlignment = Alignment.Center
) {
Text("Hello World")
}
}
Result
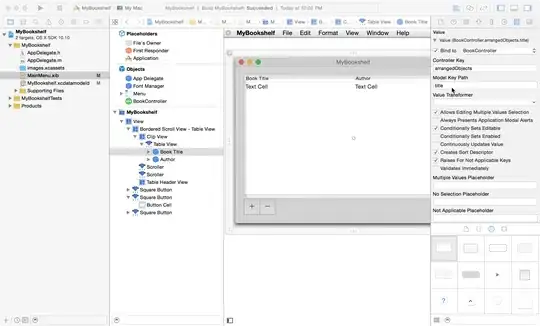