class Home extends StatefulWidget {
@override
State<Home> createState() => _HomeState();
}
class _HomeState extends State<Home> {
List<String> listData = List.generate(10, (index) => '$index');
List<String> listData2 = List.generate(5, (index) => '$index');
List<TextEditingController> listDataCTL = [];
ScrollController controler = new ScrollController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('AppBar'),
),
body: SingleChildScrollView(
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Flexible(
child: Card(
color: Colors.yellow,
child: ListView.builder(
controller: controler,
itemCount: listData.length,
shrinkWrap: true,
itemExtent: (listData.length < listData2.length) ? listData2.length / listData.length * 50 : 50,
itemBuilder: (context, index) {
return Container(
color: Colors.blue,
margin: EdgeInsets.symmetric(vertical: 4),
child: Text('Item'),
);
},
),
),
),
Flexible(
child: Card(
color: Colors.green,
child: ListView.builder(
controller: controler,
itemCount: listData2.length,
itemExtent: (listData.length > listData2.length) ? listData.length / listData2.length * 50 : 50,
shrinkWrap: true,
itemBuilder: (context, index) {
return Container(
margin: EdgeInsets.symmetric(vertical: 4),
color: Colors.red,
child: Text('Item'),
);
},
),
),
),
],
),
));
}
}
When listData.length> listData2.length
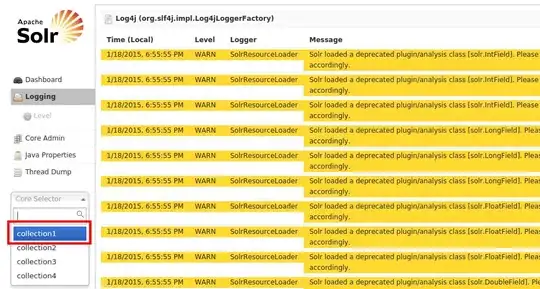
When listData2.length> listData.length
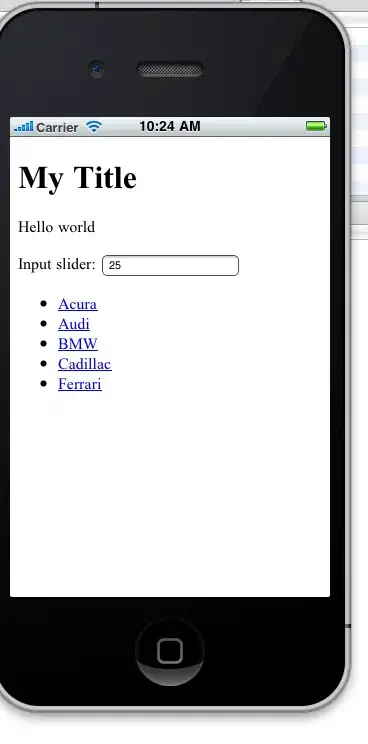
UPDATE:
Scaffold(
appBar: AppBar(
title: Text('AppBar'),
),
body: Card(
child: Row(
children: [
_buildList(list: listData, compareList: listData2),
_buildList(list: listData2, compareList: listData),
],
),
),
)
_buildList({List<String> list, List<String> compareList, double itemExtent = 50, double spacing = 8}) {
return Flexible(
child: GridView.builder(
shrinkWrap: true,
padding: EdgeInsets.all(0),
physics: const NeverScrollableScrollPhysics(),
itemCount: list.length,
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 1,
crossAxisSpacing: 0,
mainAxisSpacing:
(list.length < compareList.length) ? (((compareList.length - list.length) * itemExtent) + ((compareList.length - 1) * spacing)) / 4 : spacing,
mainAxisExtent: itemExtent,
),
itemBuilder: (context, index) {
return Container(
color: Colors.red.withOpacity(0.3 + ((index * 5) / 100)),
margin: EdgeInsets.symmetric(vertical: 0),
child: Text('Item'),
);
},
),
);
}
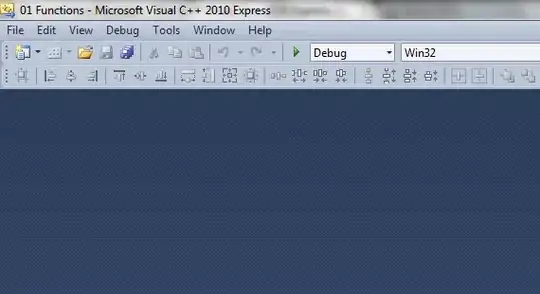
I think you want this version:
_buildList({List<String> list, List<String> compareList, double itemExtent = 50, double spacing = 8}) {
return Flexible(
child: Card(
child: ListView.builder(
itemCount: list.length,
shrinkWrap: true,
padding: EdgeInsets.only(
bottom: (list.length < compareList.length) ? (((compareList.length - list.length) * itemExtent) + ((compareList.length - 1) * 0)) : 0,
),
physics: const NeverScrollableScrollPhysics(),
itemExtent: itemExtent,
itemBuilder: (context, index) {
return Container(
color: Colors.red.withOpacity((index * 5) / 100),
margin: EdgeInsets.symmetric(vertical: 0),
child: Text('Item'),
);
},
),
),
);
}
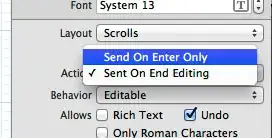