Order of Modifiers
in Composable functions is important.
Refer to this solution and other solutions in the question for more info on this.
If you want the height inside the Spacer to be the final one, you have to use Modifier.then()
.
The given code
val modifier = Modifier.fillMaxWidth().height(30.dp)
Spacer(modifier = modifier.height(5.dp))
is same as
Spacer(modifier = Modifier.fillMaxWidth().height(30.dp).height(5.dp))
But, if you change it using then()
like this
val modifier = Modifier.fillMaxWidth().height(30.dp)
Spacer(modifier = Modifier.height(5.dp).then(modifier))
It would become
Spacer(modifier = Modifier.height(5.dp).fillMaxWidth().height(30.dp))
Sample code
@Composable
fun OrderOfModifiers() {
val modifier = Modifier
.fillMaxWidth()
.height(30.dp)
Column(
modifier = Modifier
.fillMaxSize(),
) {
Spacer(modifier = modifier
.height(5.dp)
.background(DarkGray),
)
Spacer(modifier = Modifier
.height(5.dp)
.then(modifier)
.background(Cyan),
)
}
}
Sample screenshot
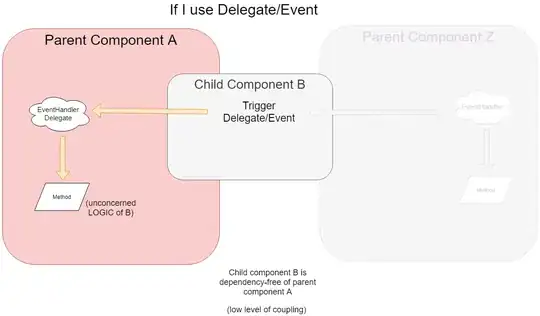