I have given the example for create the custom button and the custom label and set constraint in coding. The below code also contains the programmatically button action.
import UIKit
class ViewController: UIViewController {
let button = UIButton(frame: CGRect(x: 100,y: 400,width: 200,height: 60))
var label = UILabel(frame: CGRect(x: 100, y: 200, width: 200, height: 60))
var count : Int = 0
override func viewDidLoad() {
super.viewDidLoad()
button.setTitle("Click Button",for: .normal)
button.backgroundColor = UIColor.blue
button.setTitleColor(.white, for: .normal)
button.addTarget(self,action: #selector(buttonAction),for: .touchUpInside)
label.font = .systemFont(ofSize: 50)
label.backgroundColor = UIColor.gray
label.textAlignment = .center
self.view.addSubview(button)
self.view.addSubview(label)
}
@objc
func buttonAction() {
self.count += 1
self.label.text = "\(count)"
}
}
Output :-
Value of label increases when the button is click.
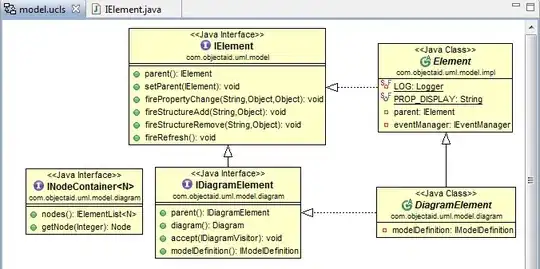