It is possible to get access to the Redis client over the Queue instance
export class SomeService {
constructor(@InjectQueue("some-queue") private someQueue: Queue) {}
async getQueueStatus(): RedisStatus {
return this.someQueue.client.status;
}
}
In this way you can access Redis client instance which has the status
property of type RedisStatus = "wait" | "reconnecting" | "connecting" | "connect" | "ready" | "close" | "end";
The queue we refer to needs to be imported into a module
BullModule.registerQueue({
name: "some-queue",
})
You can check if Redis is available at startup by the service below. Note:
- the service needs to be called in the
AppModule
- the service is rudimentary where we use a
delay
function to wait
one second before checking the RedisState
. The logic can be extended to fit your needs.
@Injectable()
export class RedisQueue {
logger = new Logger("RedisQueue");
constructor(@InjectQueue("some-queue") private someQueue: Queue) {
this.init();
}
async init() {
try {
await this.delay(1000, 1);
this.checkQueueAvailability();
} catch (e) {
this.logger.error(e);
}
}
private checkQueueAvailability(): void {
if (this.someQueue.client.status === "ready") {
this.logger.log("Redis is ready");
} else {
throw new Error("Redis not available");
}
}
delay(t: number, val: any) {
return new Promise(function (resolve) {
setTimeout(function () {
resolve(val);
}, t);
});
}
}
So when you start your app you will get -> 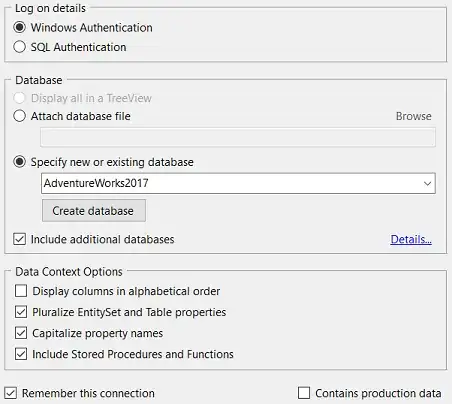
Or -> 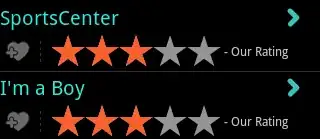