With the following toy dataframe:
import pandas as pd
df = pd.DataFrame(
{
"gadgetTypeId": [1, 2, 3, 3, 1, 2, 2, 1, 2, 1],
"created": [2017, 2018, 2018, 2019, 2017, 2018, 2017, 2017, 2019, 2019],
}
)
Here is one way to do it:
import matplotlib.pyplot as plt
plt.hist(
x=[df.loc[df["gadgetTypeId"] == i, "created"] for i in df["gadgetTypeId"].unique()],
stacked=True,
color=["r", "g", "b"],
label=df["gadgetTypeId"].unique(),
)
plt.xticks(ticks=df["created"])
plt.xlabel("created")
plt.yticks(ticks=range(df["created"].value_counts().max() + 1))
plt.ylabel("count")
plt.legend()
Which outputs:
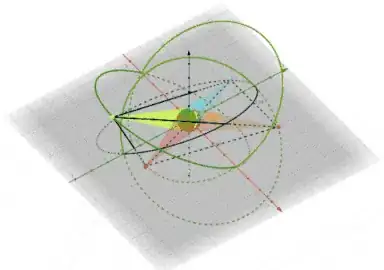
You could also consider using Seaborn, which is less verbose while better looking:
import seaborn as sns
ax = sns.histplot(
data=df,
stat="count",
multiple="stack",
x="created",
kde=False,
palette="tab10",
hue="gadgetTypeId",
element="bars",
legend=True,
)
ax.set_xticks(ticks=df["created"])
ax.set_yticks(ticks=range(df["created"].value_counts().max() + 1))
Which gets you:
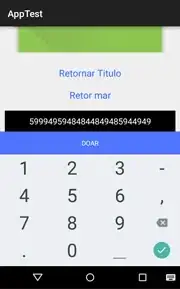