Using axios library
axios.get(
url = YOUR_URL,
data = {
headers: {
'Authorization': 'Bearer ' + access_token
}
}
);
CRUD examples
This is my demo code.
Get the access token and get query with access token.
const axios = require('axios')
const config = require('./config.json');
const getAccessToken = async () => {
try {
const resp = await axios.post(
url = 'https://id.twitch.tv/oauth2/token',
data = {
'client_id': config.CLIENT_ID,
'client_secret': config.CLIENT_SECRET,
'grant_type': 'client_credentials'
}
);
console.log(resp.data);
return resp.data.access_token;
} catch (err) {
// Handle Error Here
console.error(err);
}
};
const getQuery = async (access_token, query) => {
try {
const resp = await axios.get(
url = 'https://api.twitch.tv/helix/search/channels?query='+ query,
data = {
headers: {
'Client-Id': config.CLIENT_ID,
'Authorization': 'Bearer ' + access_token
}
}
);
console.log(resp.data);
} catch (err) {
// Handle Error Here
console.error(err);
}
};
(async () => {
const access_token = await getAccessToken();
console.log(access_token);
await getQuery(access_token, 'fruit');
})()
This is configuration file
{
"CLIENT_ID" : "thnrxxxxxxxxxxxxxqltb",
"CLIENT_SECRET" : "8yxyfxxxxxxxxxxxxxf2ztt"
}
result from terminal
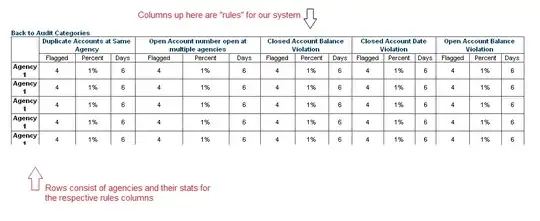
Just reference.
This method not necessary to copy an access token every time in Postman.
first step-using variable