Here a workaround, you can add the aud
as argument of the route like this:
class JWTClaims(BaseModel):
iat: int
exp: int
iss: str
sub: str
azp: str
class AccessClaims(JWTClaims):
scope: str
app = FastAPI()
# as argument it works
@app.get("/client")
async def get_user_client(aud: Optional[List[str]] = Query(None), claims:AccessClaims = Depends()):
return
And you have the aud in the swagger. It will be a list but, users can supply only one if they want.
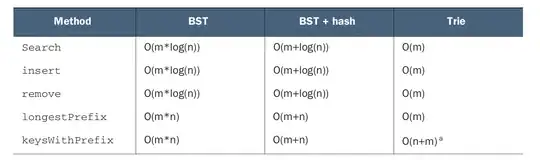
For reason I don't understand, doing this in the class doesn't work:
class JWTClaims(BaseModel):
iat: int
exp: int
aud: Optional[List[str]] = Query(None)
iss: str
sub: str
azp: str
If you're really want the Optional[str, List[str]]
, you will need to have it as a body request:
@app.get("/client")
async def get_user_client(aud: Optional[Union[str, List[str]]] = Query(None), claims:AccessClaims = Depends()):
return
leads to this error when loading the app:
async def get_user_client(aud: Optional[Union[str, List[str]]] = Query(None), claims:AccessClaims = Depends()):
File "/home/stackoverflow/lib/python3.10/site-packages/fastapi/routing.py", line 626, in decorator
self.add_api_route(
File "/home/stackoverflow/lib/python3.10/site-packages/fastapi/routing.py", line 565, in add_api_route
route = route_class(
File "/home/stackoverflow/lib/python3.10/site-packages/fastapi/routing.py", line 434, in __init__
self.dependant = get_dependant(path=self.path_format, call=self.endpoint)
File "/home/stackoverflow/lib/python3.10/site-packages/fastapi/dependencies/utils.py", line 322, in get_dependant
assert isinstance(
AssertionError: Param: aud can only be a request body, using Body()