You can use Jwt authentication to protect your web api and this is one of the method based on OAuth2.0. Here's a blog and the following codes are based on it.
OAuth2.0 is a protocol but not the implement. So you can't find samples for it. But when you searched Jwt auth
, Azure AD into .net 6
or some other products, you will find many doucuments.
Let's see some additional information which may also help you:

Let's go back to the sample, in this scenario, you have to integrate the authentication first. In .net 6, going to program.cs
and adding these code:
using Microsoft.AspNetCore.Authentication.JwtBearer;
using Microsoft.IdentityModel.Tokens;
var builder = WebApplication.CreateBuilder(args);
//adding jwt auth
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
//define which claim requires to check
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
//store the value in appsettings.json
ValidIssuer = builder.Configuration["Jwt:Issuer"],
ValidAudience = builder.Configuration["Jwt:Issuer"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(builder.Configuration["Jwt:Key"]))
};
});
...
app.UseRouting();
//adding UseAuthentication
app.UseAuthentication();
app.UseAuthorization();
In appsettings.json:
"Jwt": {
"Key": "ThisismySecretKey",
"Issuer": "Test.com"
}
Then, pls add [Authorize]
before the api controller, then you've established the authentication and when accessing the api without the correct jwt token, you will get 401 error:
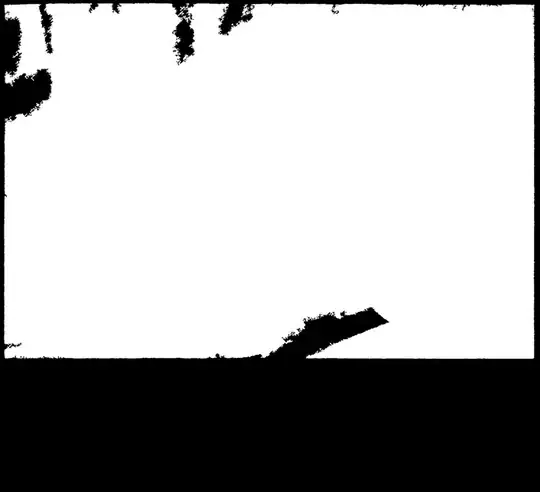
Let's generate an access token then test calling the api with the token. In another Controller without [Authorize]
, adding code like this:
using Microsoft.IdentityModel.Tokens;
using System.IdentityModel.Tokens.Jwt;
using System.Security.Claims;
private IConfiguration _config;
public HomeController(IConfiguration config)
{
_config = config;
}
public IActionResult Index()
{
ViewBag.accessToken = generateJwt();
return View();
}
private string generateJwt() {
var securityKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(_config["Jwt:Key"]));
var credentials = new SigningCredentials(securityKey, SecurityAlgorithms.HmacSha256);
//If you've had the login module, you can also use the real user information here
var claims = new[] {
new Claim(JwtRegisteredClaimNames.Sub, "user_name"),
new Claim(JwtRegisteredClaimNames.Email, "user_email"),
new Claim("DateOfJoing", "2022-09-12"),
new Claim(JwtRegisteredClaimNames.Jti, Guid.NewGuid().ToString())
};
var token = new JwtSecurityToken(_config["Jwt:Issuer"],
_config["Jwt:Issuer"],
claims,
expires: DateTime.Now.AddMinutes(120),
signingCredentials: credentials);
return new JwtSecurityTokenHandler().WriteToken(token);
}
Then calling the api with the token, you can decode the token first:
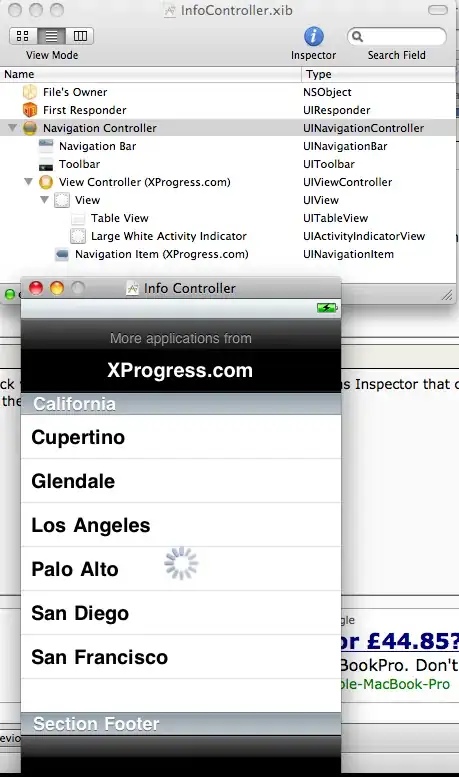