I've read through your question a few times and I'm not 'quite' sure what your goal is, but functionally creating cookies on a react app is fairly easy.
For example, I built an app that stores an authentication token in a cookie. I'll walk you through it.
I built a custom magic link auth, so I'll assume the user has clicked on a special link in their email which leads them to my /login/check
page.
const CheckPage = () => {
const router = useRouter();
const { access_key, email } = router.query;
const { data, mutate } = useLogin();
const { data: me, isLoading } = useMe();
useEffect(() => {
if (access_key && email) {
// check access key
mutate({ accessKey: access_key, email })
}
}, [access_key, email]);
useEffect(() => {
if (data?.data) createCookie(data.data.token)
}, [data])
return (
<PageContainer>
{/** show some stuff to the user*/}
</PageContainer>
)
}
Here, I grab the access_key and send that to my backend (using useLogin()) to make sure it is valid. If it is, it gets returned and stored in the data variable.
Now, I have a useEffect
function that, if the data variable exists, will create a cookie using a function I created called createCookie
.
That function looks like this...
export const createCookie = (accessToken) => {
document.cookie = `${process.env.NEXT_PUBLIC_ENVIRONMENT}_auth_access_token=${accessToken}; path=/; domain=${location.hostname}`
}
Here, I'm setting a cookie that is specific to the environment that I'm on. If I'm on a test environment, the cookie stored would like like this test_auth_access_token
and have a value of the accessToken passed in. I am also setting some additional parameters like path=/
which makes this cookie available on every page on the website, and domain=${location.hostname}
which specifies the domain at which this cookie is available (I believe this will actually get set automatically if not included).
Now, this cookie is available whenever I need to grab it (like for checking if a user has an active session, thus avoiding prompting them to login again).
If we want to grab that cookie and check its contents, we have another function that does that called getCookie
.
function getCookie(cname) {
let name = cname + "=";
let decodedCookie = decodeURIComponent(document.cookie);
let ca = decodedCookie.split(';');
for(let i = 0; i <ca.length; i++) {
let c = ca[i];
while (c.charAt(0) == ' ') {
c = c.substring(1);
}
if (c.indexOf(name) == 0) {
return c.substring(name.length, c.length);
}
}
return "";
}
which we invoke by doing something like...
const token = getCookie(`${process.env.NEXT_PUBLIC_ENVIRONMENT}_auth_access_token`)
You will know if your cookie is properly being stored by opening the inspect panel, opening the application tab, expanding the cookies option, and selecting localhost.
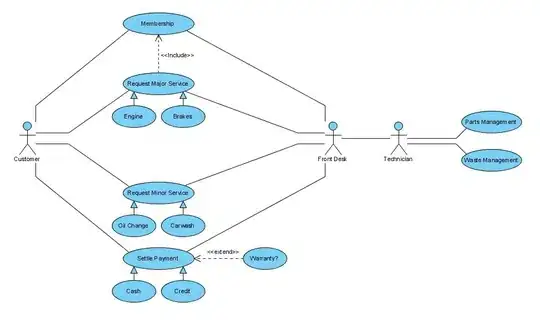

If the cookie is nonexistent or the value is incorrect, then the cookie is not being set properly and there is some other issue.
Apologies for the long post, I hope this is helpful.