It seems you have a lot of datapoints plotted so that the xticks just get overlayed due to the label font size.
If you don't need every single x-ticks displayed you can set the label locations with xticks
along with an array to display only every nth tick.
Data preparation:
Just strings for x-axis lables as an example.
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import random
import string
def random_string():
return ''.join(random.choices(string.ascii_lowercase +
string.digits, k=7))
size=1000
x_list = []
for i in range(size):
x_list.append(random_string())
y = np.random.randint(low=0, high=50, size=size)
df = pd.DataFrame(list(zip(x_list, y)),
columns =['timestamp', 'speed'])
Plot with a lot of datapoints for reference:
plt.figure(1, figsize = (20,8))
ax = sns.lineplot(data=df, x=df['timestamp'], y=df['speed'])
plt.xticks(rotation=90)
plt.title('Trip 543365 timeline', fontsize=22)
plt.ylabel('GPS speed', fontsize=18)
plt.xlabel('Timestamp', fontsize=16,)
plt.show()
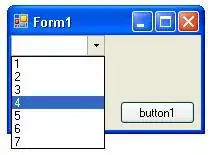
Plot with reduced xticks:
plt.figure(1, figsize = (20,8))
ax = sns.lineplot(data=df, x=df['timestamp'], y=df['speed'])
plt.xticks(rotation=90)
plt.title('Trip 543365 timeline', fontsize=22)
plt.ylabel('GPS speed', fontsize=18)
plt.xlabel('Timestamp', fontsize=16,)
every_nth_xtick = 50
plt.xticks(np.arange(0, len(x_list)+1, every_nth_xtick))
plt.show()

To cross check you can add:
print(x_list[0])
print(x_list[50])
print(x_list[100])
Just make sure it's within the same random call.