re-arrange to this:
y = x**3 - 2.381 (x**2) + (19.36/19.62)
Then seek values where y = 0
from matplotlib import pyplot as plt
import numpy as np
b = - 2.381
c = 19.36/19.62
x = np.linspace(-1, 3, num=200)
y = []
x1 = [min(x),max(x)]
y1 = [0,0]
for i in x:
y.append(i**3 + b*(i**2) + c)
fig, ax = plt.subplots()
ax.set_xlabel('x value')
ax.set_ylabel('y value')
plt.plot(x,y)
plt.plot(x1,y1)
plt.show()
which gives this:
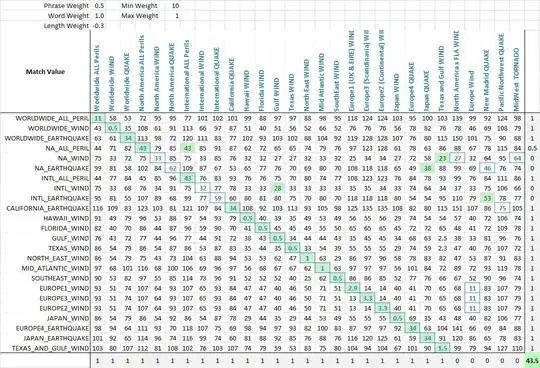
You could do newton-raphson to find the values from there or just seek values of x linearly (which is slower).