Update
If you wanted the appearance like the plot on the left instead of the plot on the right:
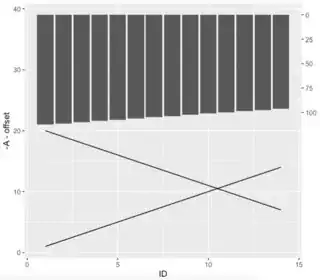
Then all you need is rev()
in geom_col
:
geom_col(aes(x = ID, y = rev(-(190-C)/5)))
Let me know if I've still misunderstood what you're looking for.
Original answer
If you want the bars to move down, they have to contain negative values.
Some of this you may already know.
When you add a second y-axis, it's really just a visual; none of your traces are actually following that axis. The 'trick' is the transformation.
If you want the bars to be above the lines as if there is a zero axis for the bars at the top and a zero axis on the bottom for the lines, some things need to change.
bars need to be negative to go down; that drives everything else
the lines need to be less than the values in the bars without losing the aspect ratio (for lack of a better term)
The easiest way to transform the y in the lines to ensure that the bars do not overlap is to offset both traces by negating the current y and offset that by the largest value that appears in the bar trace.
First, find that offset value:
offset = max((190 - Mydata$C)/5) # how much to offset the other traces
Check out what we've got so far, with the y's negated and offset:
offset = max((190 - Mydata$C)/5)
ggplot(data = Mydata) +
geom_line(aes(x = ID, y = -A - offset)) +
geom_line(aes(x = ID, y = -B - offset)) +
geom_col(aes(x = ID, y = -(190-C)/5))

Next, we need to fix both y-axes. Starting with the y-axis on the left, we need this to look like we didn't negate everything. To do that, we need to find the actual negative value that reflects the perceived zero on the y-axis.
Since column B
has the larger value (at 20), I used it to determine where the bottom is. Additionally, since the lowest value between A and B is 1, we're going to add -1 to get that zero line. To find the bottom or where we want the zero-line, find the minimum of negated column B - offset minus 1.
btm = min(-1 * Mydata$B - offset - 1) # because the min value is 1
Check out where we're at so far:
btm = min(-1 * Mydata$B - offset - 1) # because the min value of B is 1
offset = max((190 - Mydata$C)/5)
ggplot(data = Mydata) +
geom_line(aes(x = ID, y = -A - offset)) +
geom_line(aes(x = ID, y = -B - offset)) +
geom_col(aes(x = ID, y = -(190-C)/5)) +
scale_y_continuous(breaks = seq(btm, by = 10, length.out = 5),
labels = seq(0, 40, 10))
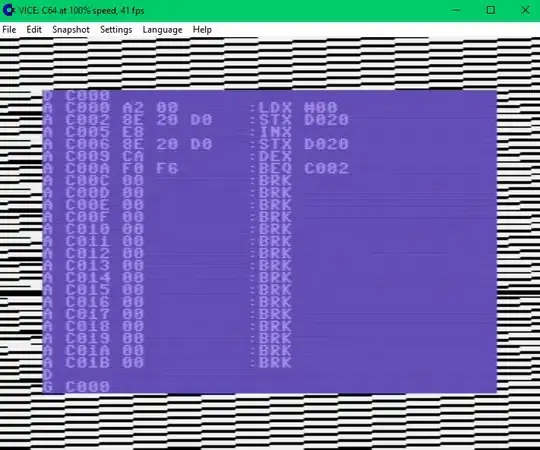
It's time to add the secondary y-axis.
I flipped the sign on the transformation and then looked at where the numbers landed on the secondary y.
btm = min(-1 * Mydata$B - offset - 1) # because the min value of B is 1
offset = max((190 - Mydata$C)/5)
ggplot(data = Mydata) +
geom_line(aes(x = ID, y = -A - offset)) +
geom_line(aes(x = ID, y = -B - offset)) +
geom_col(aes(x = ID, y = -(190-C)/5)) +
scale_y_continuous(breaks = seq(btm, by = 10, length.out = 5),
labels = seq(0, 40, 10),
sec.axis = sec_axis(~190 + . * 5)) # changed sign!
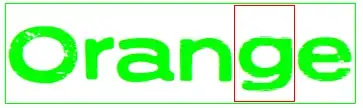
Next, we need to determine where our perceived zero is at the top on the secondary y-axis. To calculate this, we need the max of column C, when calculated as it is in the plot and transformed as the axis is transformed, then altered by the largest value of C, The largest value of B, and the offset already calculated.
top = max(((190 + (-(190 - Mydata$C)/5) * 5) + offset - btm + max(Mydata$B)))
Note that in this call to create this value:
The Y in the bars is:
-(190 - Mydata$C)/5
The Y in the bars transformed by the sec axis is:
190 + (-(190 - Mydata$C)/5) * 5
And there you go! You can adjust the labels now that it's ready however you see fit. (I'm guessing you have something in mind.)
btm = min(-1 * Mydata$B - offset - 1) # because the min value of B is 1
offset = max((190 - Mydata$C)/5)
top = max(((190 + (-(190 - Mydata$C)/5) * 5) + offset - btm + max(Mydata$B)))
ggplot(data = Mydata) +
geom_line(aes(x = ID, y = -A - offset)) +
geom_line(aes(x = ID, y = -B - offset)) +
geom_col(aes(x = ID, y = -(190-C)/5)) +
scale_y_continuous(breaks = seq(btm, by = 10, length.out = 5),
labels = seq(0, 40, 10),
sec.axis = sec_axis(~190 + . * 5, # changed sign!
breaks = seq(top - 20 * 4, top, by = 20),
labels = seq(100, 0, by = -25)))
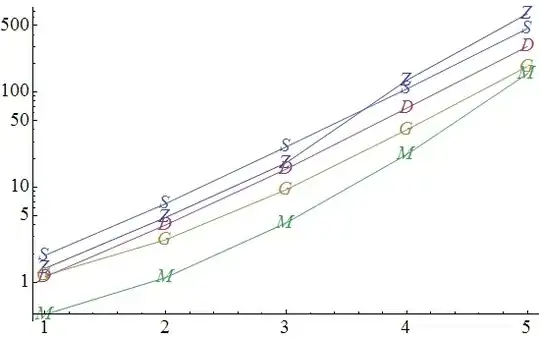