As another user pointed out, there is a difference between when you use the +
operator and the %>%
operator. One mnemonic that may work for you is that the plus sign one "adds layers" to the ggplot
, whereas the other operator is connecting code. They really do the same thing in a sense, but because ggplot is literally layering graphics in a plot, that may ensure its easier to remember (and yes, its annoying for first time R users).
I have given an example with map data below given from what looks to be the tutorial you used. First I loaded the requisite libraries after installation:
#### Load Libraries ####
library(tidyverse)
library(maps)
library(mapproj)
Then I saved the world map data into the object world
and glimpsed it to see what it is exactly, which appears to be coordinates for a world map.
#### Inspect Data
world <- map_data("world")
glimpse(world)
Rows: 99,338
Columns: 6
$ long <dbl> -69.89912, -69.89571, -69.94219, -70.00415, -70.0661…
$ lat <dbl> 12.45200, 12.42300, 12.43853, 12.50049, 12.54697, 12…
$ group <dbl> 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2…
$ order <int> 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 13, 14, 15, 16, 1…
$ region <chr> "Aruba", "Aruba", "Aruba", "Aruba", "Aruba", "Aruba"…
$ subregion <chr> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, …
Then I created the map as a saved object worldmap
using the pipes discussed. First, I just used the +
operator to make it easy to see that they are only chaining the plot layers on top of each other. There is no need for a pipe here.
#### Create World Map ####
worldmap <- ggplot(world,
aes(x = long,
y = lat,
group = group)) +
geom_path() +
scale_y_continuous(breaks = (-2:2) * 30) +
scale_x_continuous(breaks = (-4:4) * 45)
worldmap
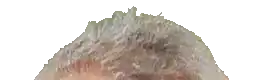
Now I will make a very small change to show the difference. The world
data was included into the ggplot
command last time. This time I'm going to pipe it into the ggplot, which is what the %>% operator does...it simply takes the data and then says "and then use the command after".
worldmap <- world %>%
ggplot(aes(x = long,
y = lat,
group = group)) +
geom_path() +
scale_y_continuous(breaks = (-2:2) * 30) +
scale_x_continuous(breaks = (-4:4) * 45)
worldmap
And you get the exact same thing! So just remember the +
operator is only for ggplot
. Anything else that gets chained together in R uses the %>%
pipe (when its possible to do so). For your example, it seems you are also missing the ggplot
function and the variables needed to complete it. I have added an example here:
world %>%
ggplot(aes(x=long,
y=lat))+
coord_map("orthographic",
orientation = c(39, -98, 0))
Which gives you this:
