We can convert the image Blob URL to a based64 encoded string like the below, and then
function getScreenShotImageBase64(viewer, width, height) {
return new Promise((resolve) => {
viewer.getScreenShot(width, height, blobURL => {
let img = new Image();
let tmpCanvas = document.createElement('canvas');
let ctx = tmpCanvas.getContext('2d');
tmpCanvas.width = width;
tmpCanvas.height = height;
img.onload = function() {
// draw viewer image on canvas
ctx.drawImage(img, 0, 0, width, height);
resolve(tmpCanvas.toDataURL('image/png'));
};
img.src = blobURL;
});
});
}
let imageBase64 = await getScreenShotImageBase64(viewer, viewer.container.offsetWidth, viewer.container.offsetHeight);
Here is the preview of the result:
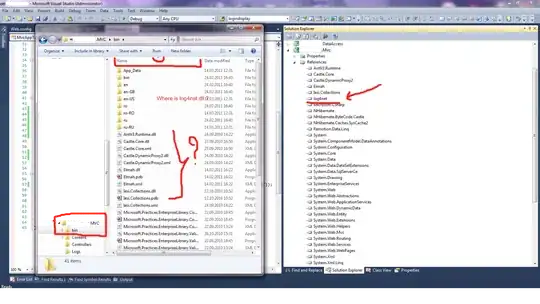
Afterward, send the base64 string to your backend server, and then you can either save the image base64 string to your database,
Or resave the base64 string to file. For example in nodejs.
fs.writeFileSync(path.join(uploadPath, fileName), new Buffer(base64, 'base64'))
ref: How to upload image using Base64 string in NodeJs