I could not find a solution to the Original question. There is no way to break a website outside of the mobile app browser. But I did come up with a solution to warn the user of the potential issue so that they open it in their native mobile browser.
A very basic Angular detection service
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class BrowserDetectService {
// https://raw.githubusercontent.com/3rd-Eden/useragent/master/lib/regexps.js
fbWvRegex = [
new RegExp("\\[(FBAN/MessengerForiOS|FB_IAB/MESSENGER);FBAV/(\\d+)(?:\\.(\\d+)(?:\\.(\\d+)|)|)"),
new RegExp("\\[FB.*;(FBAV)/(\\d+)(?:\\.(\\d+)|)(?:\\.(\\d+)|)"),
new RegExp("\\[FB.*;"),
];
snapWebRegex = [
new RegExp("(Snapchat)\\/(\\d+)\\.(\\d+)\\.(\\d+).(\\d+)"),
new RegExp("(Snapchat)(\\d+)\\.(\\d+)\\.(\\d+).(\\d+)"),
];
AndroidRegex = [
new RegExp("(Android)"),
];
iPhoneRegex = [
new RegExp("^(.*)-iPad\\/(\\d+)(?:\\.(\\d+)|)(?:\\.(\\d+)|)(?:\\.(\\d+)|) CFNetwork"),
new RegExp("^(.*)-iPhone/(\\d+)(?:\\.(\\d+)|)(?:\\.(\\d+)|)(?:\\.(\\d+)|) CFNetwork"),
new RegExp("(iPod|iPhone|iPad).+GSA/(\\d+)\\.(\\d+)\\.(\\d+) Mobile"),
new RegExp("(iPod|iPhone|iPad).+Version/(\\d+)\\.(\\d+)(?:\\.(\\d+)|).*[ +]Safari"),
new RegExp("(iPod|iPod touch|iPhone|iPad);.*CPU.*OS[ +](\\d+)_(\\d+)(?:_(\\d+)|).* AppleNews\\/\\d+\\.\\d+\\.\\d+?"),
new RegExp("(iPod|iPhone|iPad).+Version/(\\d+)\\.(\\d+)(?:\\.(\\d+)|)"),
new RegExp("(iPod|iPod touch|iPhone|iPad);.*CPU.*OS[ +](\\d+)_(\\d+)(?:_(\\d+)|).*Mobile.*[ +]Safari"),
new RegExp("(iPod|iPod touch|iPhone|iPad);.*CPU.*OS[ +](\\d+)_(\\d+)(?:_(\\d+)|).*Mobile"),
new RegExp("(iPod|iPhone|iPad).* Safari"),
new RegExp("(iPod|iPhone|iPad)"),
new RegExp("(Outlook-iOS)/\\d+\\.\\d+\\.prod\\.iphone \\((\\d+)\\.(\\d+)\\.(\\d+)\\)"),
];
constructor(
) { }
detectWebView() : string | undefined {
const userAgent = navigator.userAgent;
if (this.matchesAny(userAgent, this.fbWvRegex)) {
return 'Facebook';
}
if (this.matchesAny(userAgent, this.snapWebRegex)) {
return 'Snapchat';
}
return undefined;
}
detectMobileOS(): string | undefined {
const userAgent = navigator.userAgent;
if (this.matchesAny(userAgent, this.AndroidRegex)) {
return 'Android';
}
if (this.matchesAny(userAgent, this.iPhoneRegex)) {
return 'iPhone';
}
return undefined;
}
matchesAny(userAgent: string, parsers: RegExp[]): boolean {
let res: any;
for (let i = 0; i < parsers.length; i++) {
if (parsers[i].exec(userAgent)) {
return true;
}
}
return false;
}
fixInstructionsImgUrl(): string | undefined {
const app = this.detectWebView();
const os = this.detectMobileOS();
if (!app) { return undefined; }
if (os === 'Android') {
if (app === 'Facebook') {
return '/HowTo-OpenInChrome-Android-Facebook.png';
} else { // Snapchat is last remaining OS with image help
return '/HowTo-OpenInChrome-Android-Snapchat.png';
}
} else { // iOS
// we only have one image for now on iOS
// if (app === 'Snapchat') {
return '/HowTo-OpenInChrome-iOS-Snapchat.png';
// }
}
// return '/HowTo-OpenInChrome.png';
// Add whichever apps and OS images you have here
}
}
Then I used this in the usual way from a component to conditionally show/hide content. The result is:
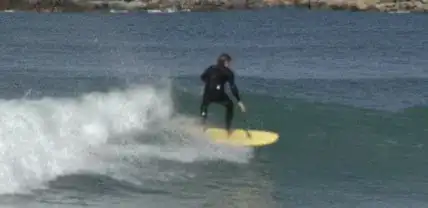
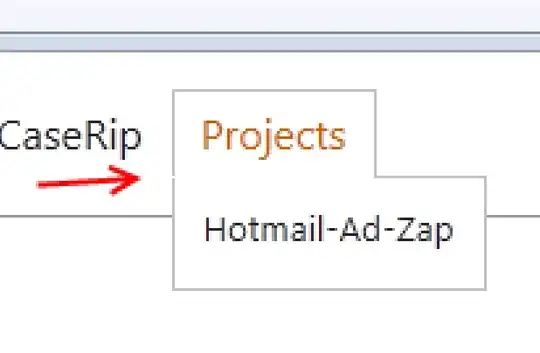