Can I publish the API somewhere for free for now to debug and test the
entire web app?
You don't need to publish your API
to test from your frontend app
. You can can do that running your Web Api
project locally therefore, setting debugger pointer to any break point.
Example Debugging Using Swagger In Localhost:
You could have a look on following example, I am testing the Web API
project running on local environment
using Swagger
. I am sending request to my desired API
and debugging at the same time whether the response expected. See below screenshot:
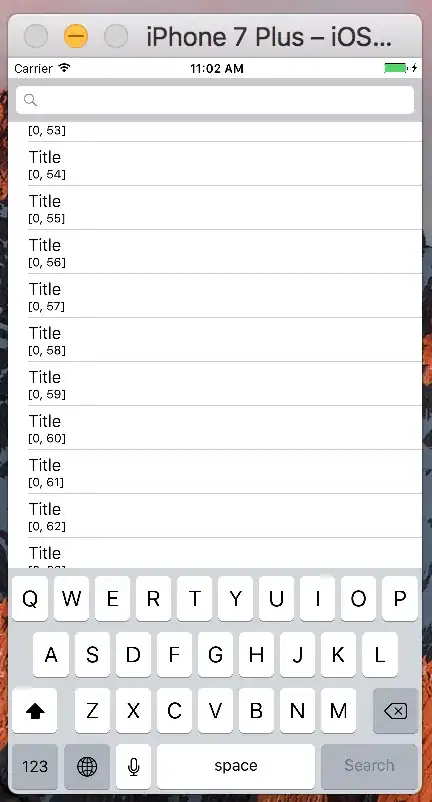
What if you encounter "Origin 'localhost:<port>' has been blocked by CORS policy"
Error :
You could simply handel above error by following ways:
If you want to allow all request Endpoint:
builder.UseCors(x => x
.AllowAnyMethod()
.AllowAnyHeader()
.SetIsOriginAllowed(origin => true) // allow any origin
Note: Above code resolve your CORS
error and allow all coming request from your frontend app
If you want to allow Certain request Endpoint:
var MyAllowSpecificOrigins = "_myAllowSpecificOrigins";
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddCors(options =>
{
options.AddPolicy(name: MyAllowSpecificOrigins,
policy =>
{
policy.WithOrigins("http://localhost:5094");
});
});
Then use as below:
app.UseCors(MyAllowSpecificOrigins);
Note: Above code allow the request only from the endpoint containing http://localhost:5094
. URL
If you sitll need further information you could refer to our official document here