Red color is split in the cicular region of the HSV color space, so there is red part in 0+x and a red part in 360-x (180-x/2 in opencv because the 360° are divided by 2 there to fit into 8 bit).
For example 0+15 and 180-15 (+/-10 is also ok) in opencv give good results in your example:
int main()
{
cv::Mat img = cv::imread("C:/data/StackOverflow/redSign.jpg");
cv::Mat hsv;
cv::cvtColor(img, hsv, cv::COLOR_BGR2HSV);
cv::Scalar low_red1(165, 50, 5);
cv::Scalar high_red1(180, 255, 255);
cv::Scalar low_red2(0, 50, 5);
cv::Scalar high_red2(15, 255, 255);
cv::Mat mask1;
cv::Mat mask2;
cv::inRange(hsv, low_red1, high_red1, mask1);
cv::inRange(hsv, low_red2, high_red2, mask2);
cv::imshow("mask1", mask1);
cv::imshow("mask2", mask2);
cv::imshow("mask1+mask2", mask1 + mask2);
cv::waitKey(0);
}
Giving this result:
Mask1:
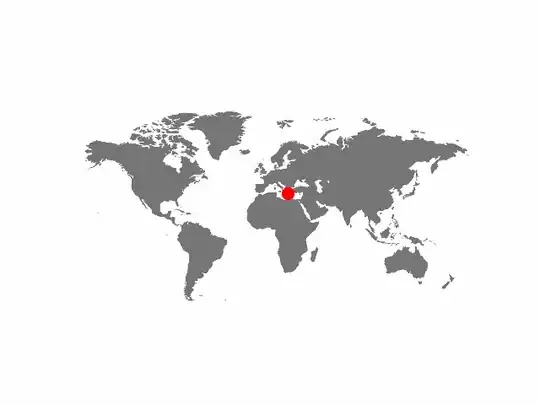
Mask2:
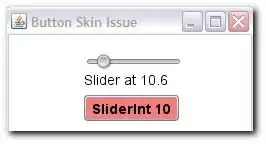
Mask total:
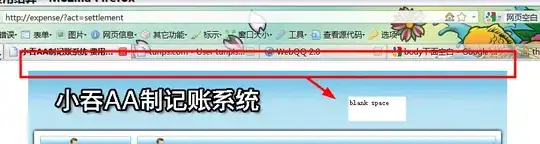
If you want to segment red with a single inRange call you can shift the hue channel, but that's likely not more efficient, but might make it easier to write some kind of color segmentation general code:
Have a look at the "shiftedH" part of: OpenCV Edge/Border detection based on color