Luckily Inno Setup gives enough abilities to build own forms so you can imitate any page of uninstall form on another modal form.
Here's what I managed to create. The form takes dimensions from UninstallForm
, contains a TNewNotebook
control and buttons to jump between pages and to cancel the dialog.
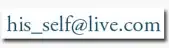
const
ControlGap = 5; // determined empirically
// Set Back/Next buttons state according to current selected notebook page
procedure UpdateButtonsState(Form: TSetupForm);
var
Notebook: TNewNotebook;
BtnBack, BtnNext: TButton;
begin
Notebook := TNewNotebook(Form.FindComponent('Notebook'));
BtnBack := TButton(Form.FindComponent('BtnBack'));
BtnNext := TButton(Form.FindComponent('BtnNext'));
// Update buttons state
BtnBack.Enabled := (Notebook.ActivePage <> Notebook.Pages[0]);
if Notebook.ActivePage <> Notebook.Pages[Notebook.PageCount - 1] then
begin
BtnNext.Caption := SetupMessage(msgButtonNext)
BtnNext.ModalResult := mrNone;
end
else
begin
BtnNext.Caption := SetupMessage(msgButtonFinish);
BtnNext.ModalResult := mrYes;
end;
end;
// Change notebook page
procedure BtnPageChangeClick(Sender: TObject);
var
NextPage: TNewNotebookPage;
Notebook: TNewNotebook;
Form: TWinControl;
Button, BtnBack, BtnNext: TButton;
begin
Button := TButton(Sender);
Form := Button;
while not (Form is TSetupForm) do
Form := Form.Parent;
Notebook := TNewNotebook(Form.FindComponent('Notebook'));
BtnBack := TButton(Form.FindComponent('BtnBack'));
BtnNext := TButton(Form.FindComponent('BtnNext'));
// Avoid cycled style of Notebook's page looping
if (Button = BtnBack) and (Notebook.ActivePage = Notebook.Pages[0]) then
NextPage := nil
else
if (Button = BtnNext) and (Notebook.ActivePage = Notebook.Pages[Notebook.PageCount - 1]) then
NextPage := nil
else
NextPage := Notebook.FindNextPage(Notebook.ActivePage, Button = BtnNext);
Notebook.ActivePage := NextPage;
UpdateButtonsState(TSetupForm(Form));
end;
// Add a new page to notebook and return it
function AddPage(NotebookForm: TSetupForm): TNewNotebookPage;
var
Notebook: TNewNotebook;
begin
Notebook := TNewNotebook(NotebookForm.FindComponent('Notebook'));
Result := TNewNotebookPage.Create(Notebook);
Result.Notebook:=Notebook;
Result.Parent:=Notebook;
Result.Align := alClient;
if Notebook.ActivePage = nil then
Notebook.ActivePage := Result;
UpdateButtonsState(NotebookForm);
end;
// Create a form with notebook and 3 buttons: Back, Next, Cancel
function CreateNotebookForm: TSetupForm;
var
Notebook: TNewNotebook;
NotebookPage: TNewNotebookPage;
Pan: TPanel;
TmpLabel: TLabel;
MaxWidth, i: Integer;
BtnBack, BtnNext, BtnCancel: TButton;
BtnLabelMsgIDs: array of TSetupMessageID;
begin
Result := CreateCustomForm;
Result.SetBounds(0, 0, UninstallProgressForm.Width, UninstallProgressForm.Height);
Result.Position := poOwnerFormCenter;
Notebook := TNewNotebook.Create(Result);
Notebook.Parent := Result;
Notebook.Name := 'Notebook'; // will be used for searching
Notebook.Align := alClient;
Pan := TPanel.Create(Result);
Pan.Parent := Notebook;
Pan.Caption := '';
Pan.Align := alBottom;
// Create buttons
BtnNext := TNewButton.Create(Result);
with BtnNext do
begin
Parent := Pan;
Name := 'BtnNext'; // will be used for searching
Caption := SetupMessage(msgButtonNext);
OnClick := @BtnPageChangeClick;
ParentFont := True;
end;
BtnBack := TNewButton.Create(Result);
with BtnBack do
begin
Parent := Pan;
Caption := SetupMessage(msgButtonBack);
Name := 'BtnBack'; // will be used for searching
OnClick := @BtnPageChangeClick;
ParentFont := True;
end;
BtnCancel := TNewButton.Create(Result);
with BtnCancel do
begin
Parent := Pan;
Name := 'BtnCancel'; // will be used for searching
Caption := SetupMessage(msgButtonCancel);
ModalResult := mrCancel;
Cancel := True;
ParentFont := True;
end;
// Determine dimensions of buttons. Should use TCanvas.TextWidth here
// but it doesn't allow Font property assignment :(
TmpLabel := TLabel.Create(Result);
with TmpLabel do
begin
Left := 0;
Top := 0;
Parent := Pan;
ParentFont := True;
Visible := False;
WordWrap := False;
Autosize := True;
end;
// Determine max label width among these labels: Back, Next, Cancel, Finish
SetArrayLength(BtnLabelMsgIDs, 4);
BtnLabelMsgIDs[0] := msgButtonBack;
BtnLabelMsgIDs[1] := msgButtonNext;
BtnLabelMsgIDs[2] := msgButtonCancel;
BtnLabelMsgIDs[3] := msgButtonFinish;
MaxWidth := 0;
for i := Low(BtnLabelMsgIDs) to High(BtnLabelMsgIDs) do
begin
TmpLabel.Caption := SetupMessage(BtnLabelMsgIDs[i]) + 'WWW'; // Add letters for surrounding spaces
if MaxWidth < TmpLabel.Width then
MaxWidth := TmpLabel.Width;
end;
TmpLabel.Caption := 'Yy'; // Determine height
// Assign sizes and positions
Pan.ClientHeight := TmpLabel.Height*4;
with BtnBack do
begin
Width := MaxWidth;
Height := TmpLabel.Height*2;
Left := Parent.ClientWidth - 3*(MaxWidth + ScaleX(ControlGap));
Top := (Parent.ClientHeight - Height) div 2;
end;
with BtnNext do
begin
Width := MaxWidth;
Height := TmpLabel.Height*2;
Left := Parent.ClientWidth - 2*(MaxWidth + ScaleX(ControlGap));
Top := (Parent.ClientHeight - Height) div 2;
end;
with BtnCancel do
begin
Width := MaxWidth;
Height := TmpLabel.Height*2;
Left := Parent.ClientWidth - 1*(MaxWidth + ScaleX(ControlGap));
Top := (Parent.ClientHeight - Height) div 2;
end;
end;
Usage is like
// UninstallProgressForm is about to be shown
// Show modal dialog which allows to select additional components to uninstall
procedure InitializeUninstallProgressForm;
var
Form: TSetupForm;
i: Integer;
NotebookPage: TNewNotebookPage;
ModResult: Integer;
begin
Form := CreateNotebookForm;
for i := 1 to 4 do
begin
NotebookPage := AddPage(Form);
with NotebookPage do
begin
Color := clWindow;
with TLabel.Create(Form) do
begin
Parent := NotebookPage;
SetBounds(0, 0, 50, 30);
Autosize := true;
Font.Size := 14;
Caption := 'Label ' + IntToStr(i);
end;
end;
end;
ModResult := Form.ShowModal;
if ModResult = mrYes then
MsgBox('Continuing uninstall', mbInformation, MB_OK)
else
begin
MsgBox('Cancelled', mbInformation, MB_OK);
Abort;
end;
...
end;