First, get your df
into a shape that is easier to digest for plotting methods, using df.pivot
:
import pandas as pd
from yahooquery import Ticker
tickers = Ticker('aapl nflx', asynchronous=True)
df = tickers.history()
df_pivot = df.reset_index().pivot(index='date',
columns='symbol', values='adjclose')
symbol aapl nflx
date
2022-01-03 181.259918 597.369995
2022-01-04 178.959457 591.150024
2022-01-05 174.199142 567.520020
2022-01-06 171.291199 553.289978
2022-01-07 171.460495 541.059998
Next, have a look at sns.lineplot
:
import matplotlib.pyplot as plt
import matplotlib.dates as md
import matplotlib.ticker as mtick
import seaborn as sns
sns.set_theme()
fig, ax = plt.subplots(figsize=(10,6))
ax = sns.lineplot(data=df_pivot, palette=['r','b'], dashes=False)
# adjust axes for readability
ax.xaxis.set_major_locator(md.WeekdayLocator(byweekday = 1))
ax.set_xlim(df_pivot.index.min(), df_pivot.index.max())
ax.yaxis.set_major_locator(mtick.MultipleLocator(50))
plt.xticks(rotation=90)
plt.tight_layout()
plt.show()
Result:
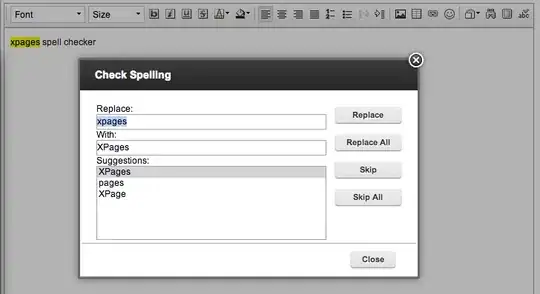
Incidentally, if you want to compare both stocks, it may make more sense to plot the percentages. E.g.:
df_pivot_perc = df_pivot.div(df_pivot.iloc[0,:]).mul(100)
fig, ax = plt.subplots(figsize=(10,6))
ax = sns.lineplot(data=df_pivot_perc, palette=['r','b'], dashes=False)
ax.xaxis.set_major_locator(md.WeekdayLocator(byweekday = 1))
ax.set_xlim(df_pivot.index.min(), df_pivot.index.max())
fmt = '%.0f%%'
yticks = mtick.FormatStrFormatter(fmt)
ax.yaxis.set_major_formatter(yticks)
ax.yaxis.set_major_locator(mtick.MultipleLocator(10))
plt.xticks(rotation=90)
plt.tight_layout()
plt.show()
Result:
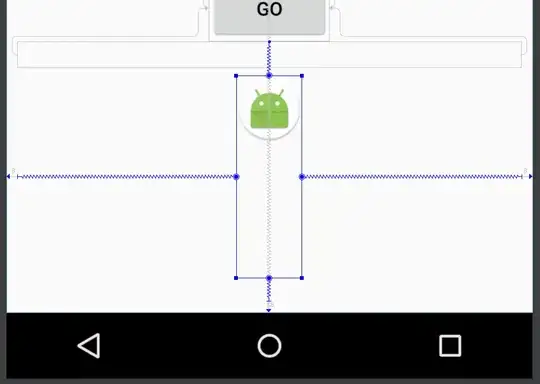